CSS Advanced
The box model
In the default box model, when you set the width or height of an element, you are specifying the width or
height of its content; any padding, border, and margins are then added to that width.
We can adjust the box model behavior with its box sizing property.
- box-sizing: content-box (by default) any height or width you specify only sets the size of the content box.
- box-sizing: border-box the height and width properties set the combined size of the content, padding, and border.
Top
<-----------Left----------->
Margin
Border
Padding
Content
<-----------Width----------->
<-----------Height----------->
<-----------Right----------->
Bottom
CSS layouts
Float
Floats are the oldest method for laying out a web.
A float pulls an element (often an image) to one side of its
container, allowing the document flow to wrap around it.
If you float multiple elements in the same direction, they will
stack alongside one anothe
Floats are still the only way to move an image to the side of the
page and allow text to wrap around it.
HTML
<img class="Float_Img" src="SRC/IMAGES/Oran.jpg"/>
<p> Lorem, ipsum dolor sit amet consectetur adipisicing elit. Accusamus rem ad aliquid vero, cumque eligendi quasi impedit ipsam quos voluptas aperiam reiciendis placeat, cum recusandae sapiente. Ullam iure laudantium modi!
</p>
CSS
.Float_Img {
float: left;
}
Result
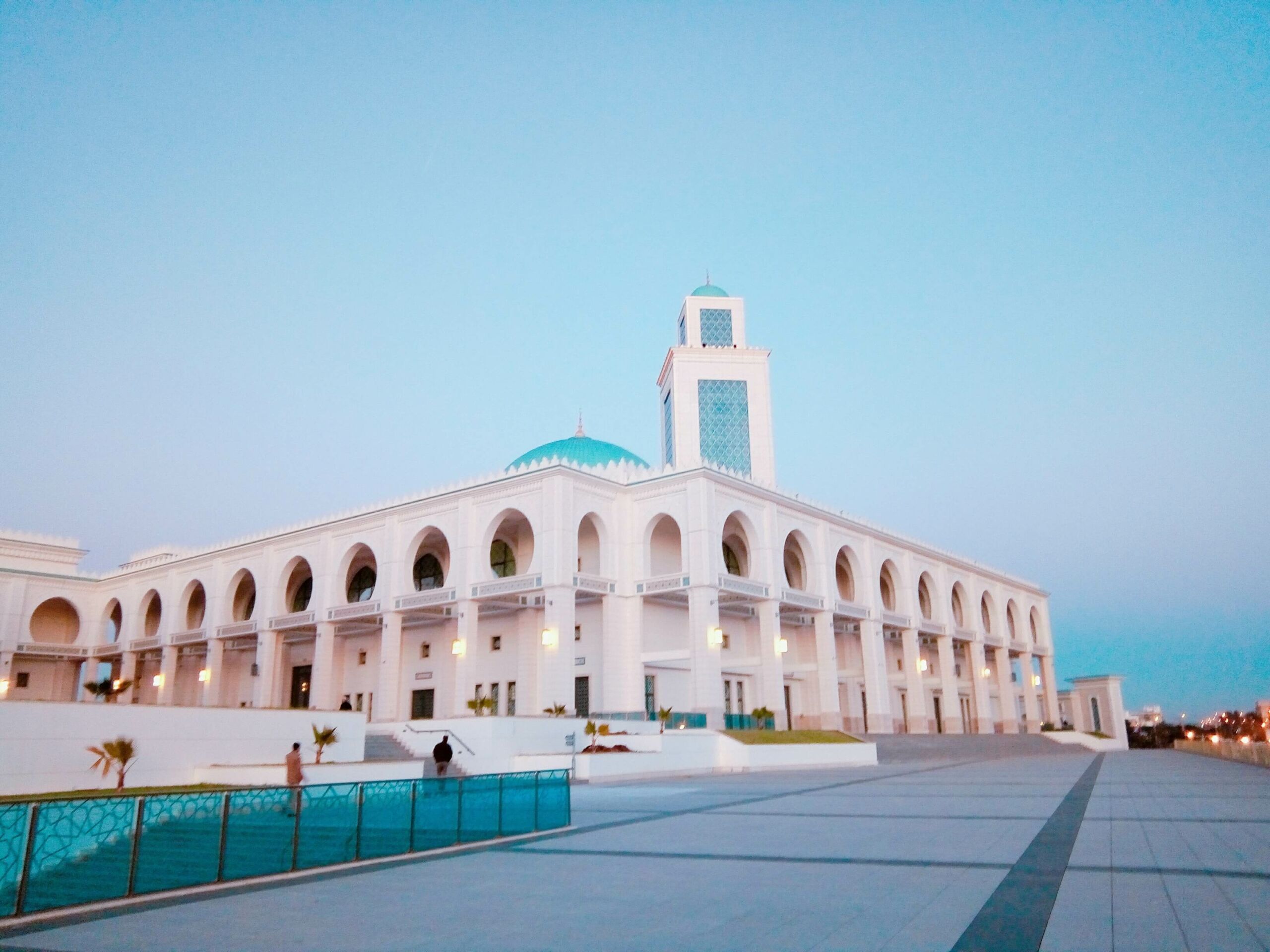
Lorem, ipsum dolor sit amet consectetur adipisicing elit. Vero labore vel temporibus quia fuga quod, voluptatem totam quidem pariatur velit earum voluptates, iusto porro, natus sapiente repudiandae? Impedit, libero delectus. Lorem ipsum dolor sit amet consectetur adipisicing elit. Deserunt reiciendis cumque nam consequatur illum veritatis doloribus aspernatur. Cupiditate perferendis, porro quod distinctio veritatis vitae fugiat odit explicabo, exercitationem alias aut. Lorem ipsum dolor sit amet, consectetur adipisicing elit.
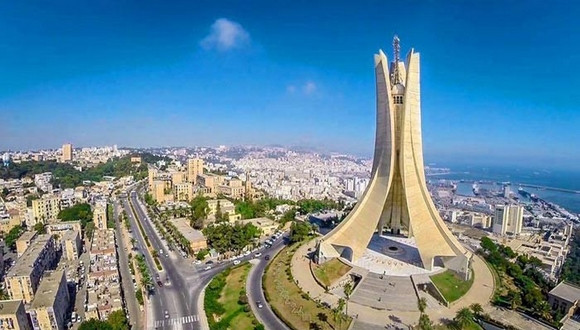
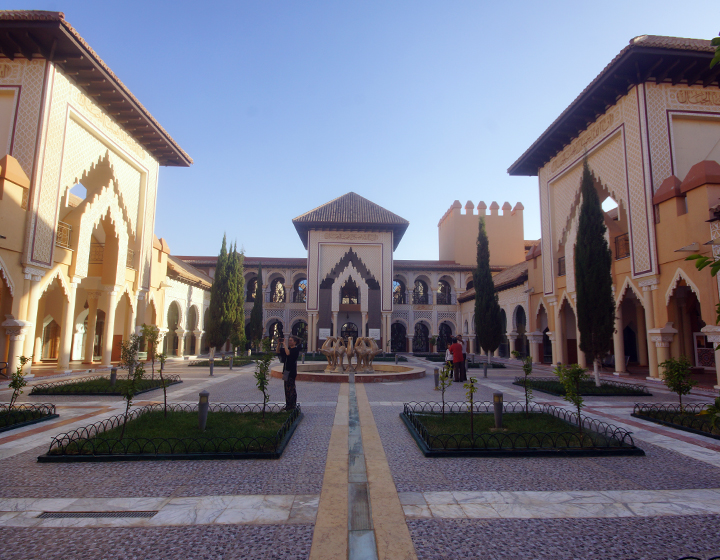
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Minima, perspiciatis labore consequuntur tenetur velit numquam odit doloribus dolor mollitia laborum facere optio aperiam magnam, unde vel dolores accusamus. Nihil, harum?. Lorem ipsum dolor sit amet, consectetur adipisicing elit. Minima, perspiciatis labore consequuntur tenetur velit numquam odit doloribus dolor mollitia laborum facere optio aperiam magnam, unde vel dolores accusamus. Nihil, harum? Lorem ipsum dolor sit amet, consectetur adipisicing elit.
FlexBox
Flexbox—formally Flexible Box Layout—is a new method for laying out
elements on the page It introduces 12 new properties to CSS,
including some shorthand properties.
Flexbox begins with the familiar display property. Applying display: flex to an element
turns it into a flex container, and its direct children turn into
flex items.
By default, flex items align side by side, left to
right, all in one row. The flex container fills the available width
like a block element, but the flex items may not necessarily fill
the width of their flex container.
The flex items are all the same
height, determined naturally by their contents. You can also use
display: inline-flex.
Flex direction
This specifies the direction of the main axis. The cross axis will be perpendicular to the main axis.
<-----------Main axis----------->
<------Cross axis------>
HTML
<div class="Flex_Container">
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
.Flex_Container {
display: flex;
flex-direction: row;
}
Result
flex-direction: row (default)
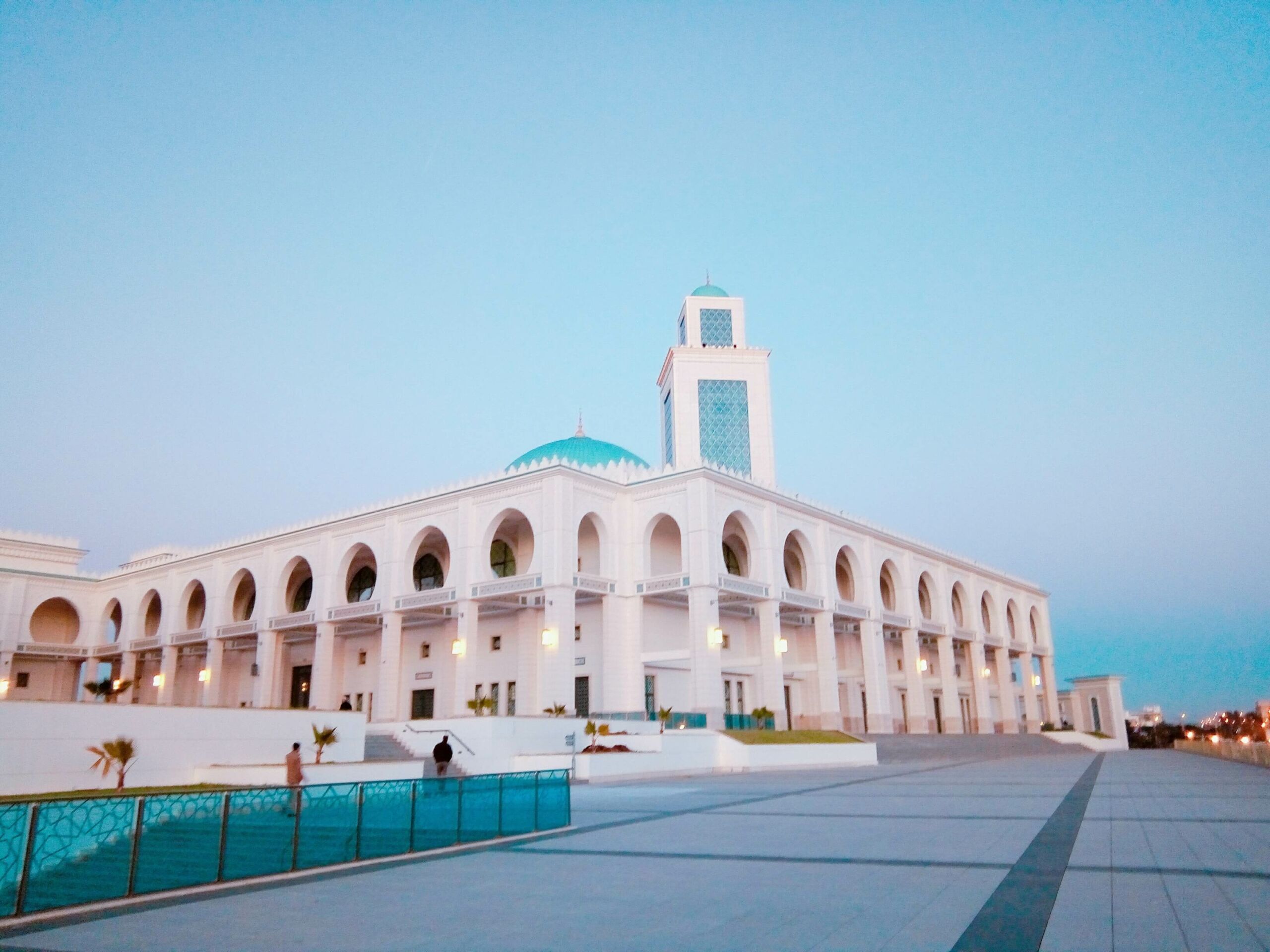
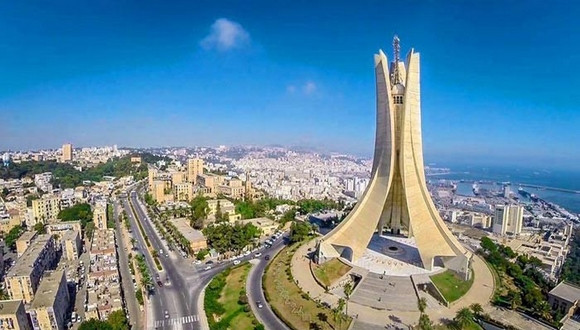
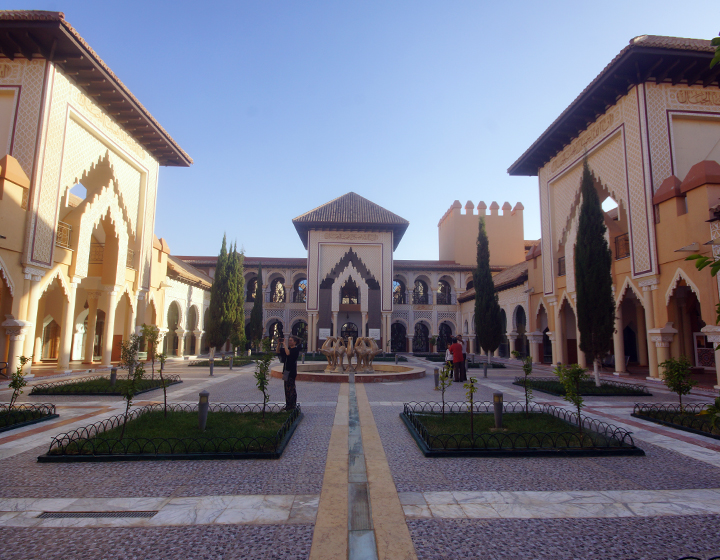
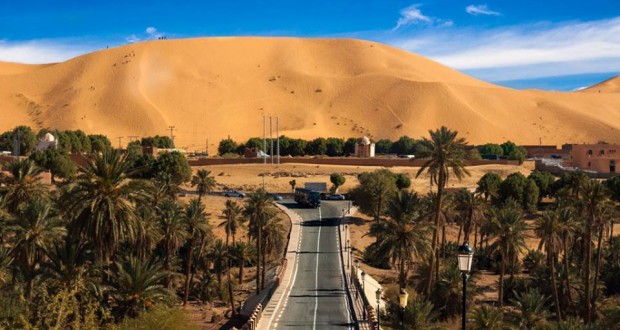
flex-direction: row-reverse
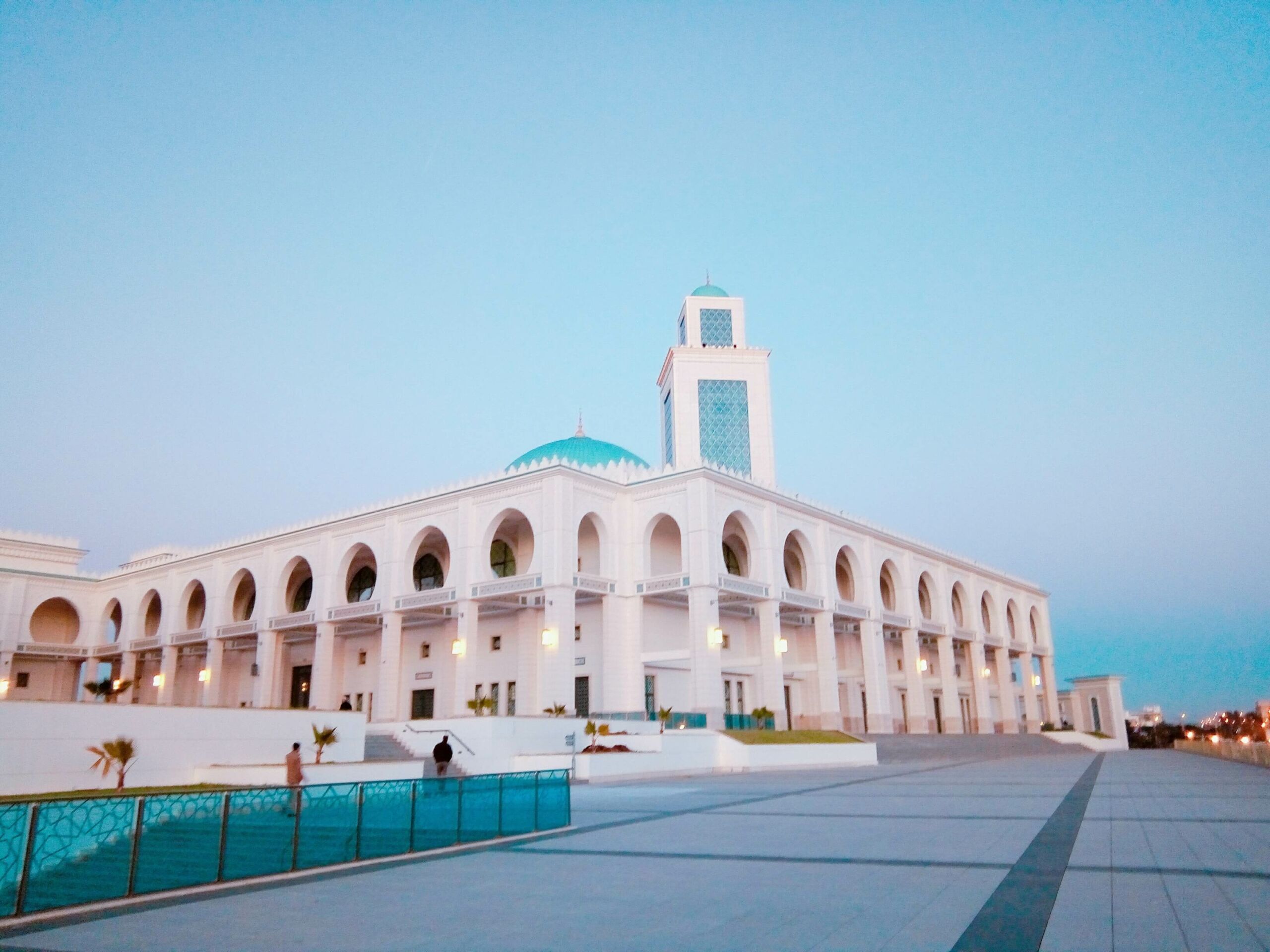
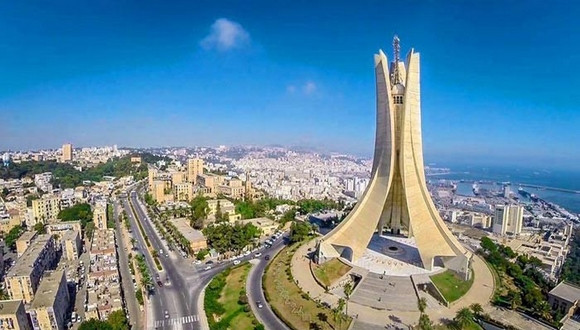
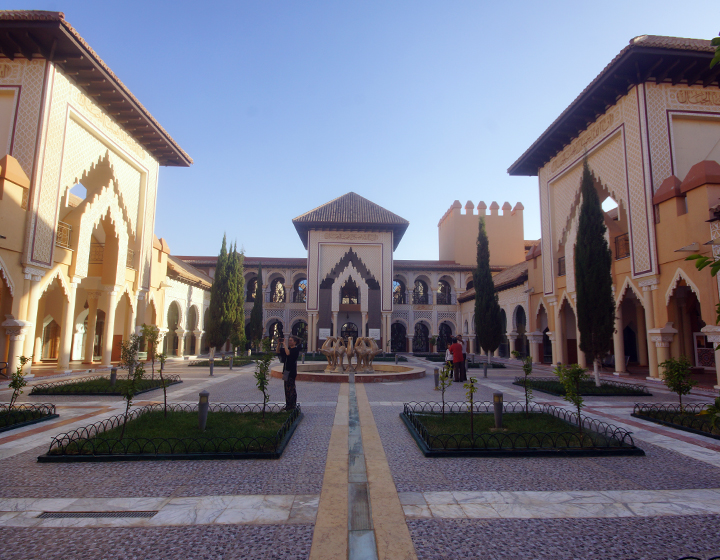
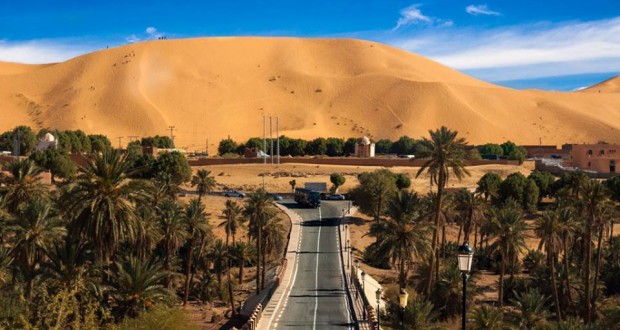
HTML
<div class="Flex_Container">
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
.Flex_Container {
display: flex;
flex-direction: column;
}
Result
flex-direction: column
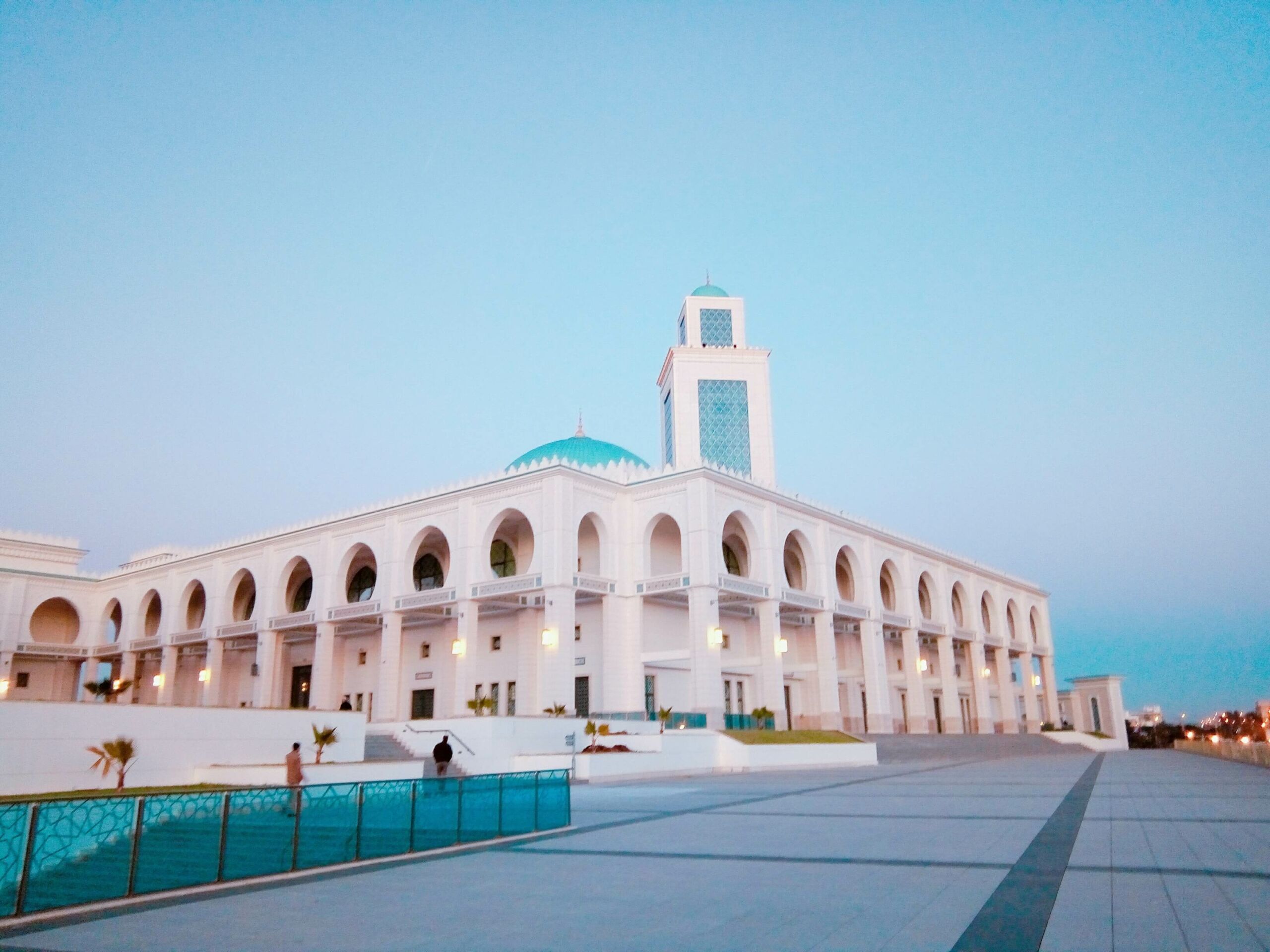
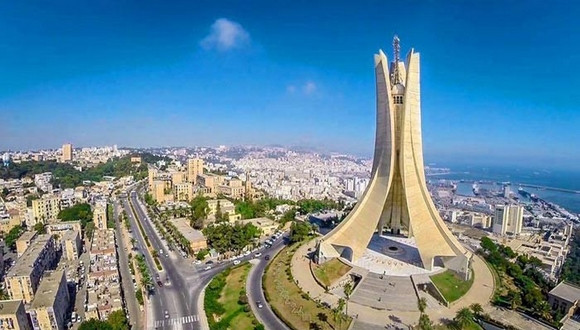
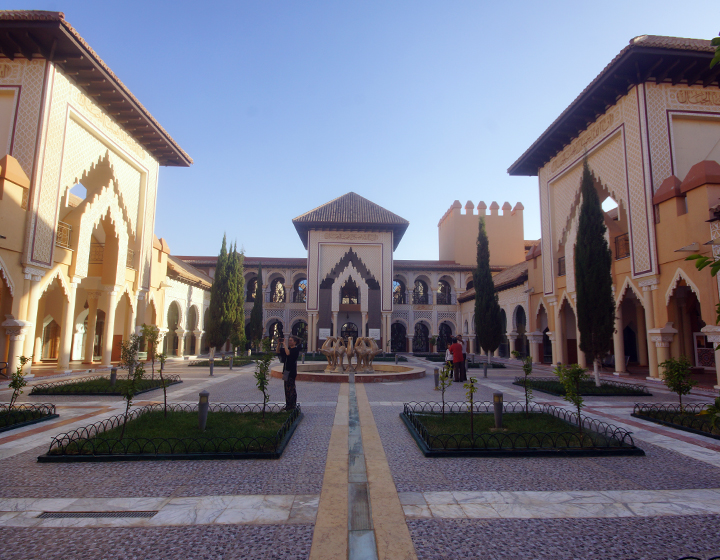
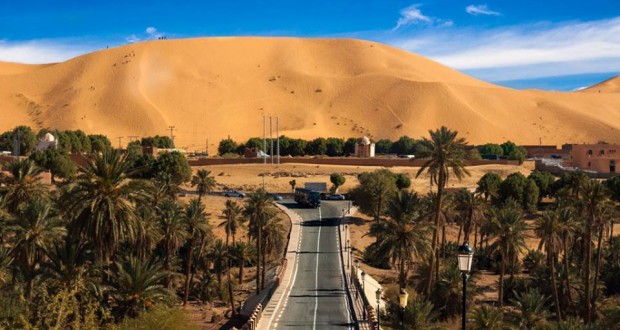
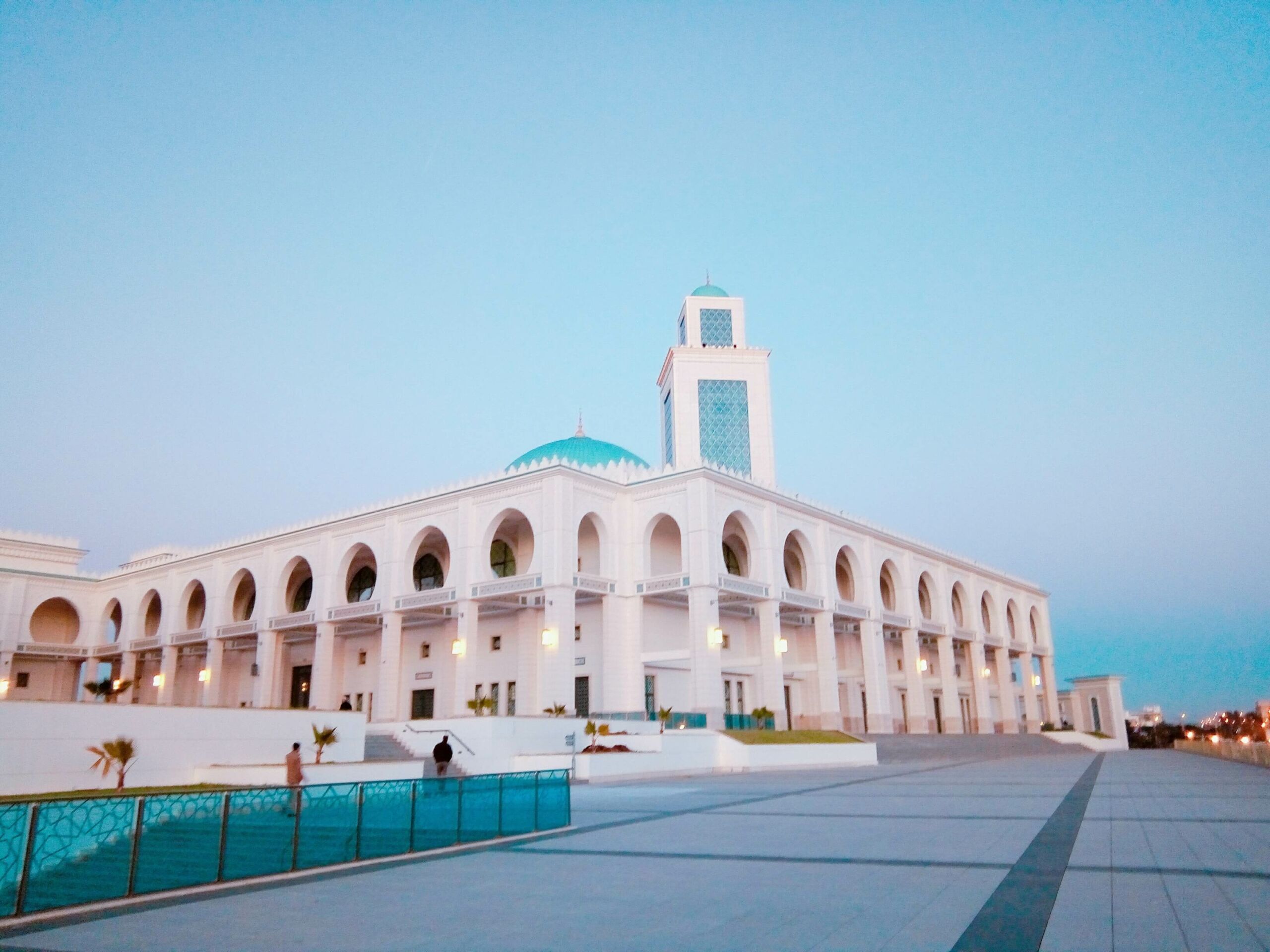
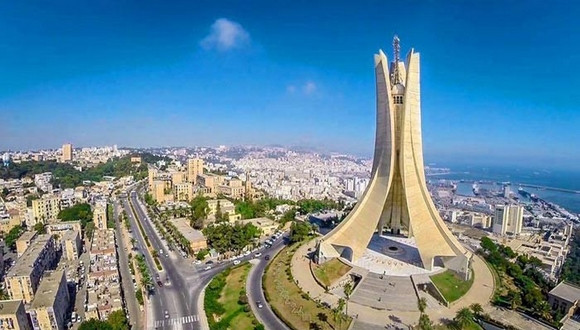
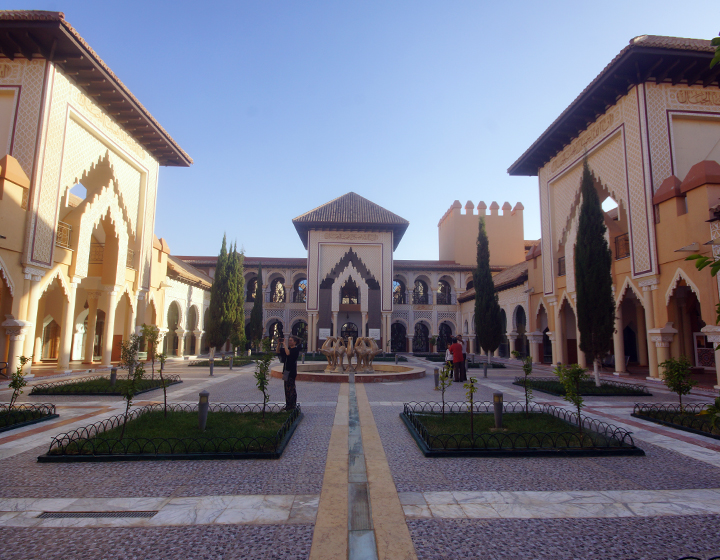
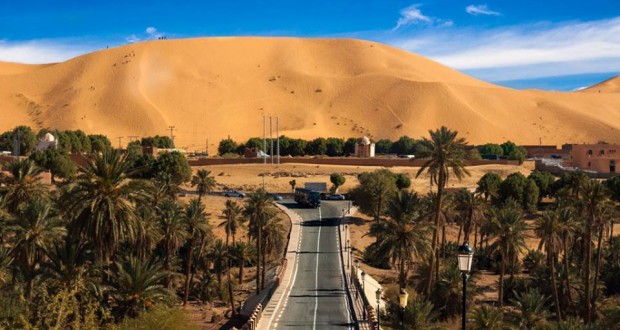
flex-direction: column-reverse
Flex wrap
This specifies whether flex items will wrap on to a new row inside
the flex container (or on to a new column if flex-direction is
column or column-reverse).
Also flex-flow is a shorthand for flex-direction flex-wrap
HTML
<div class="Flex_Container">
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
.Flex_Container {
display: flex;
flex-wrap: nowrap;
}
Result
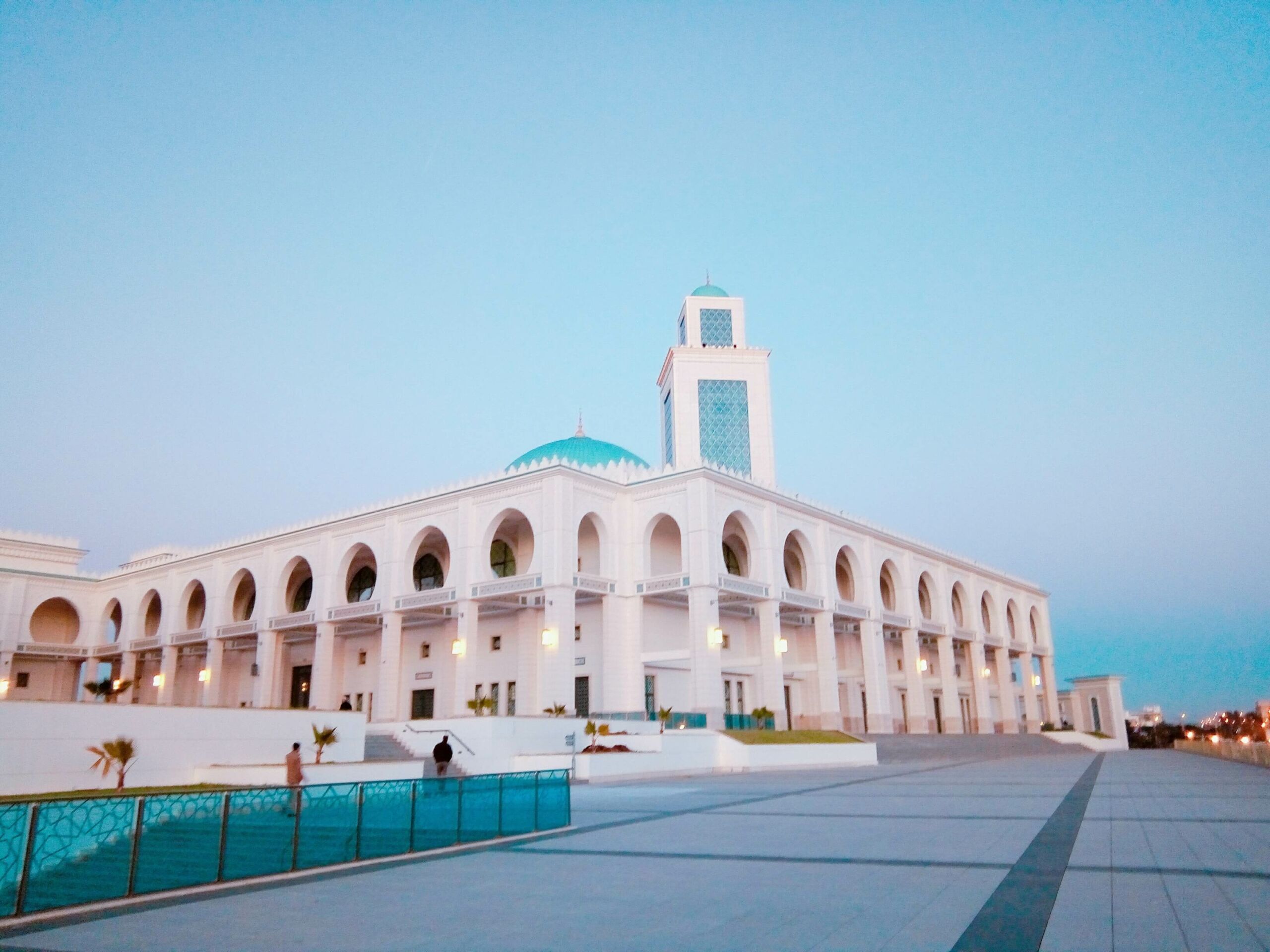
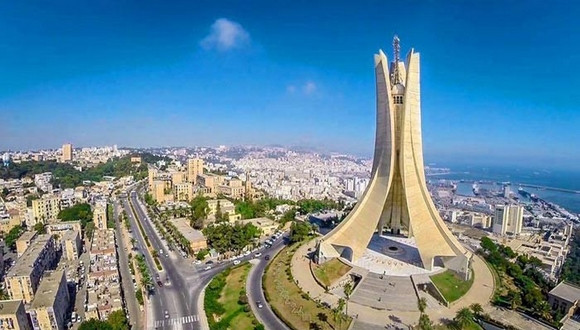
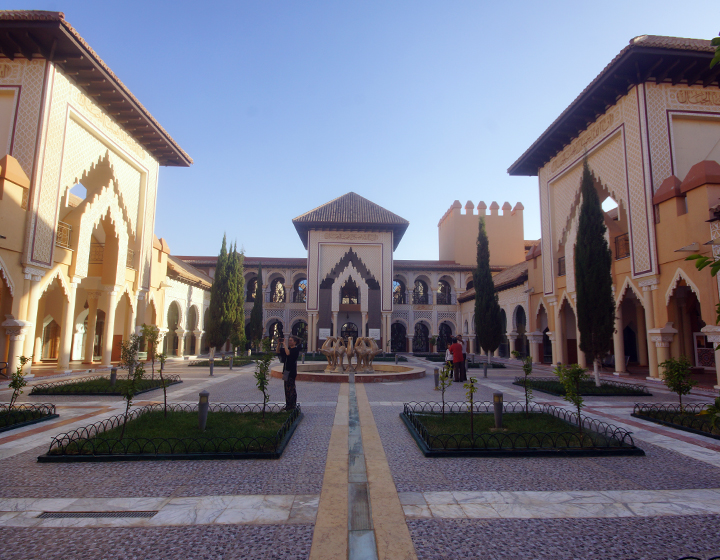
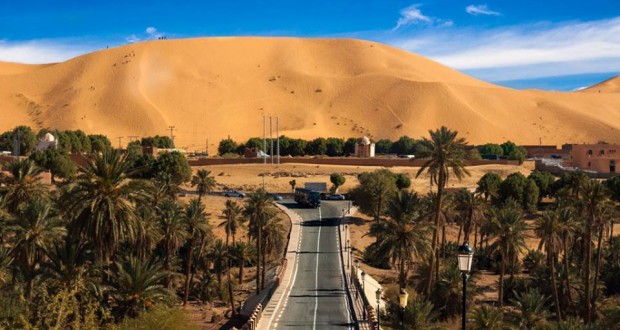
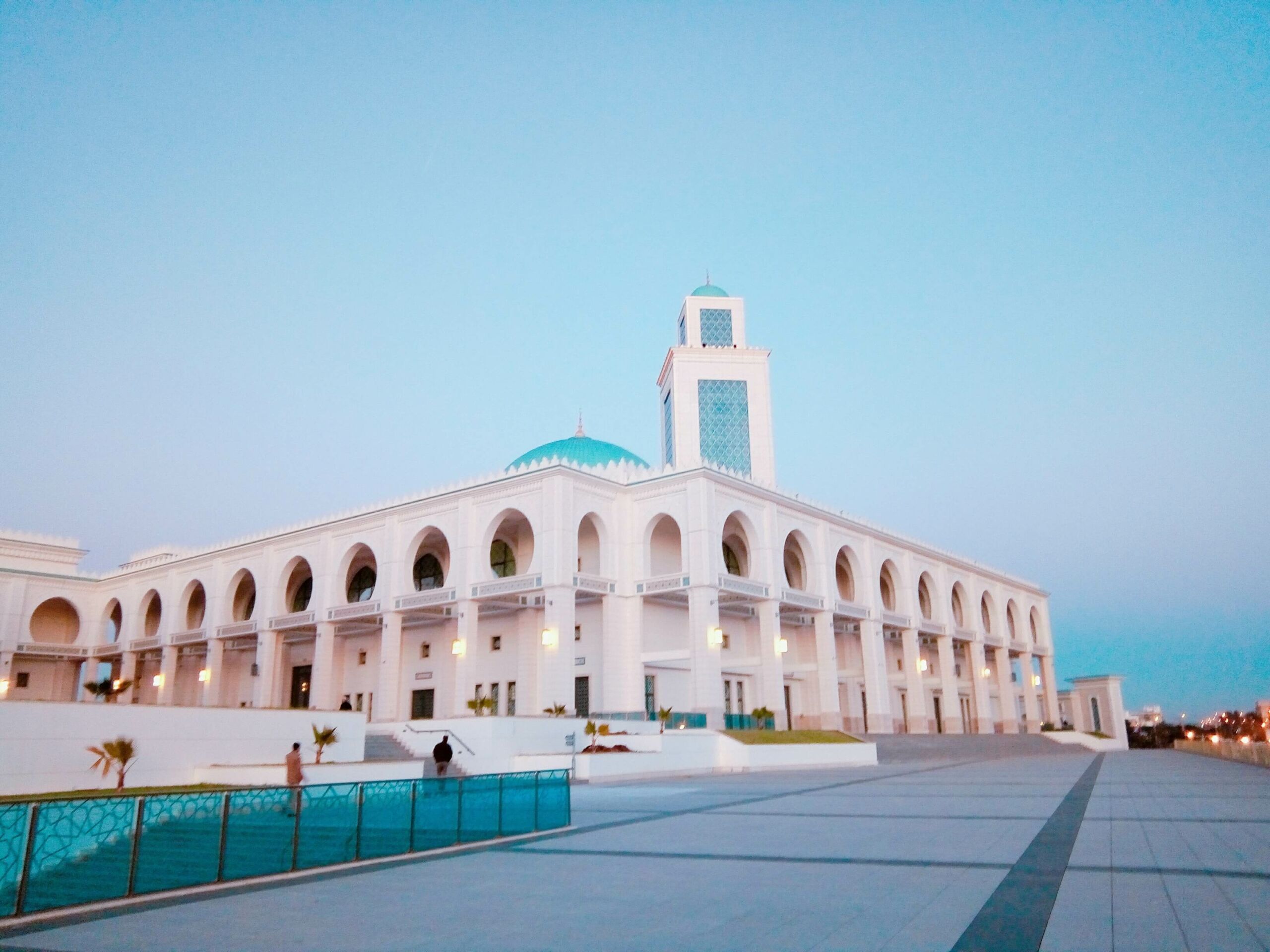
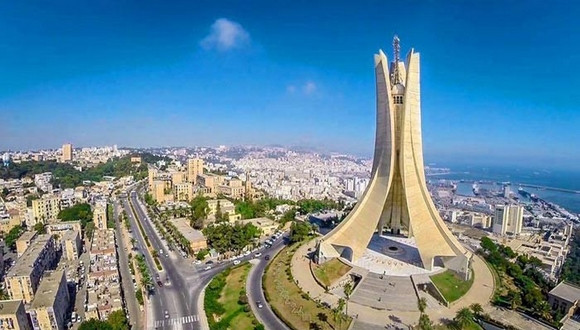
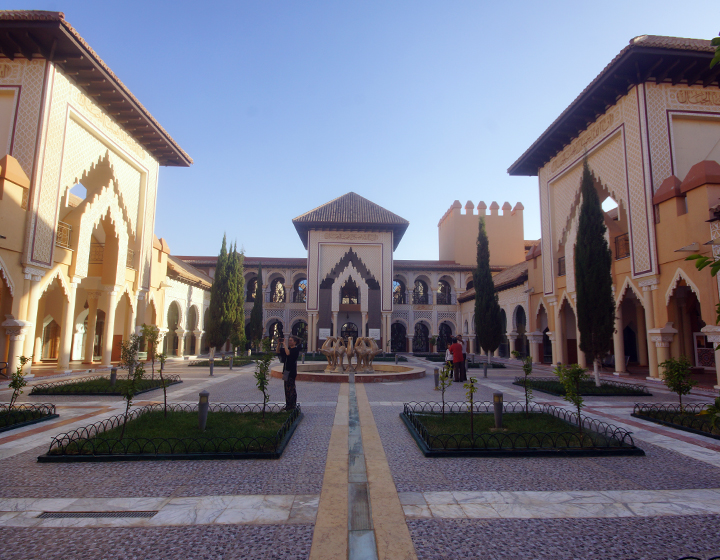
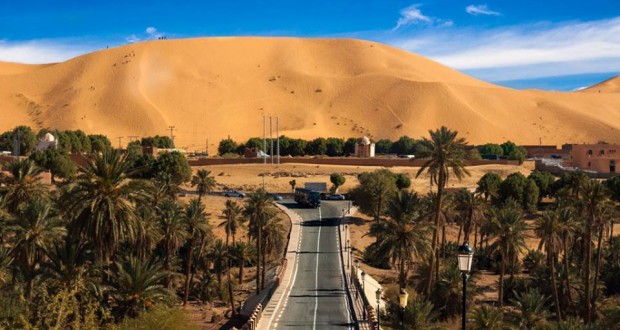
flex-wrap: nowrap
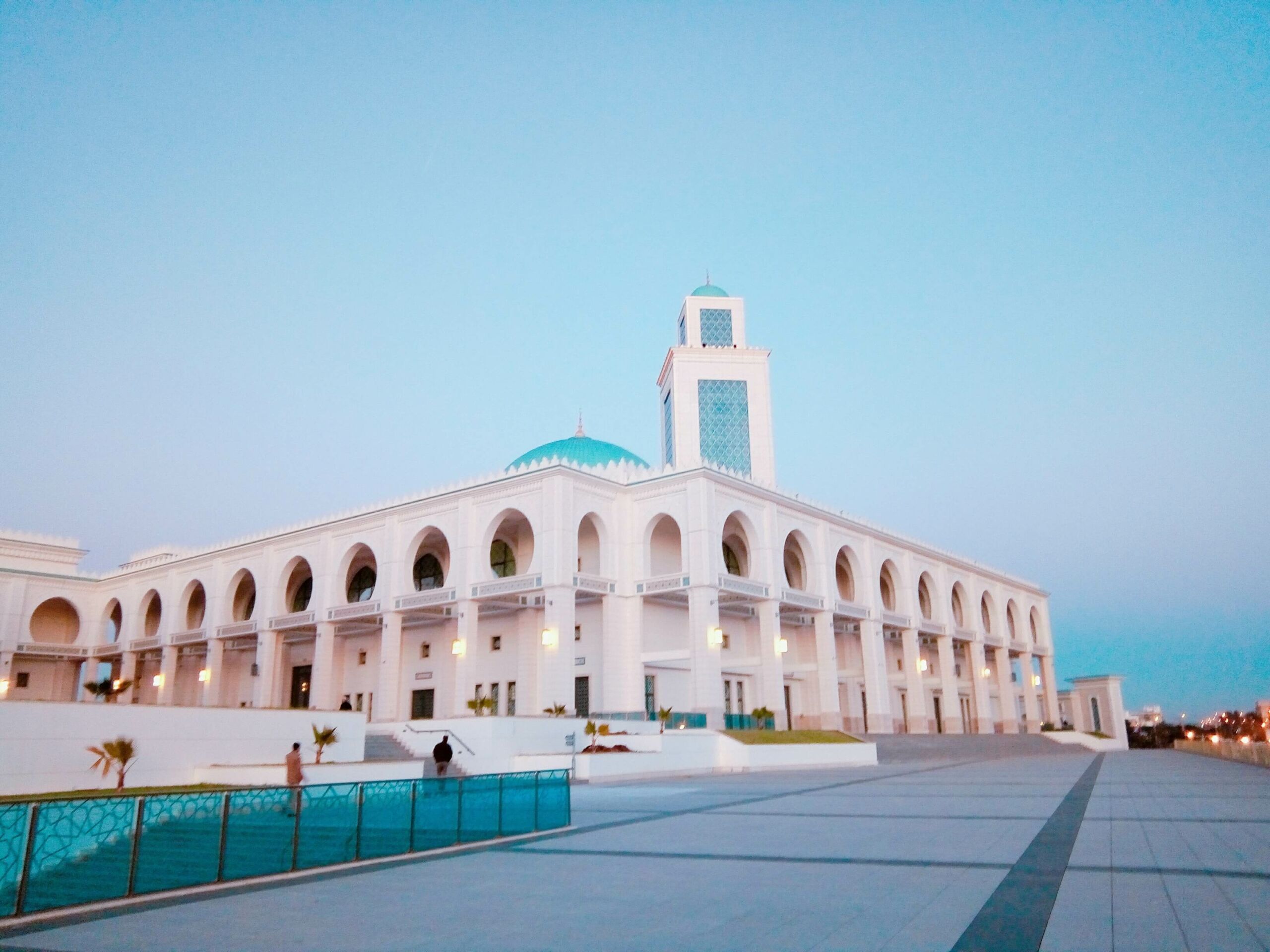
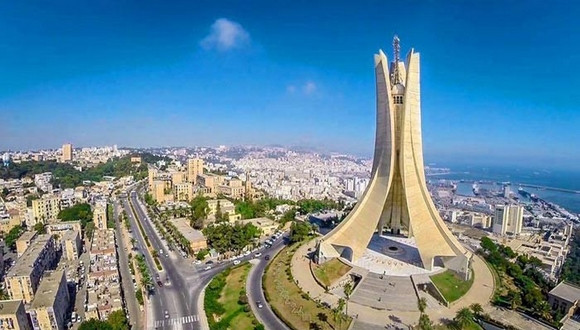
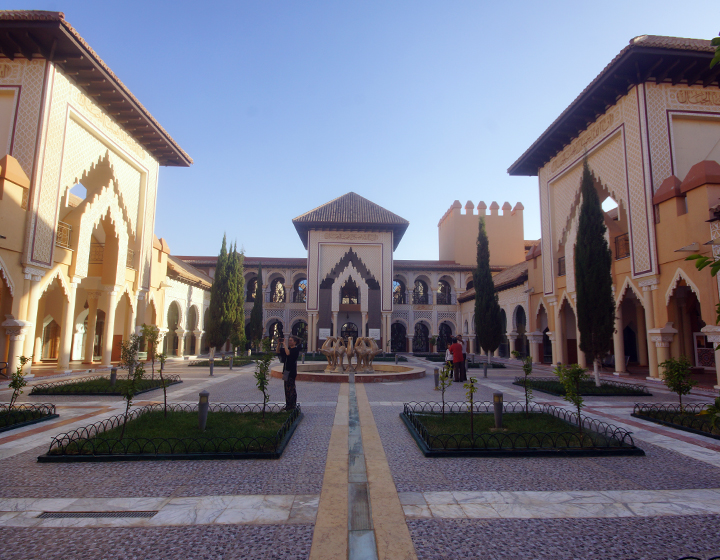
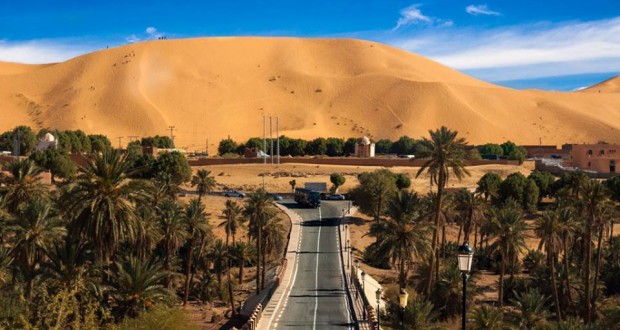
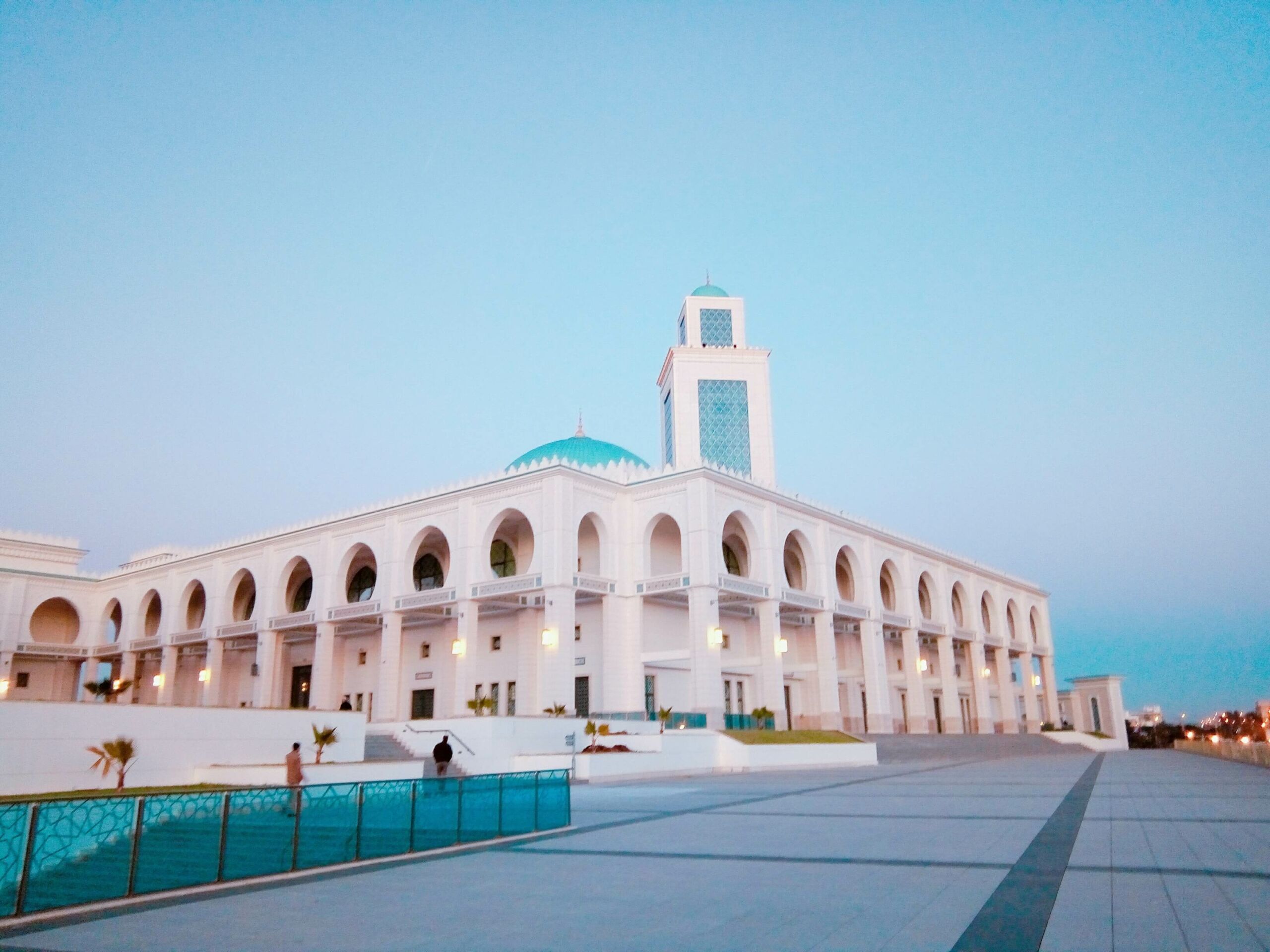
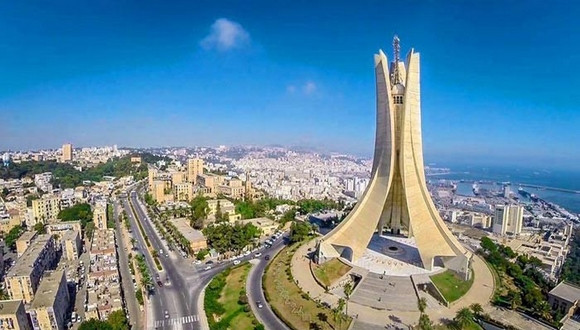
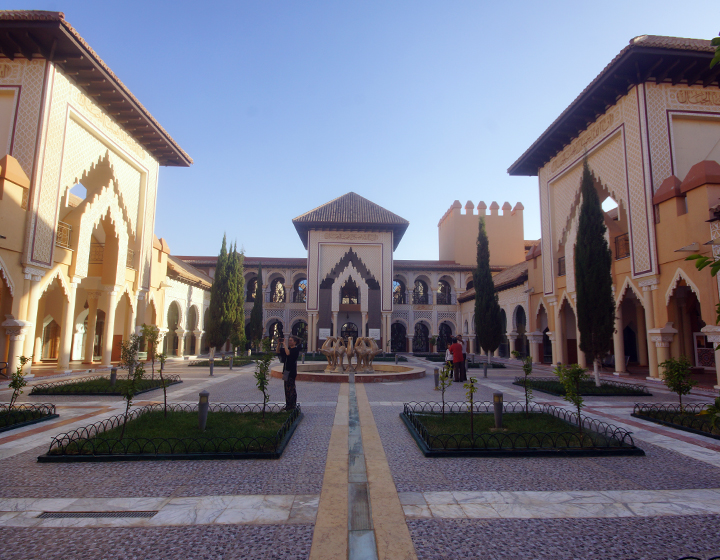
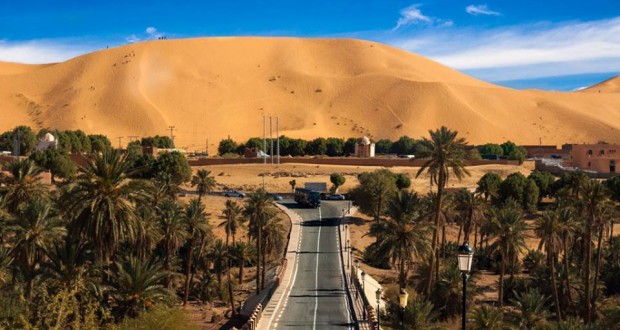
flex-wrap: wrap
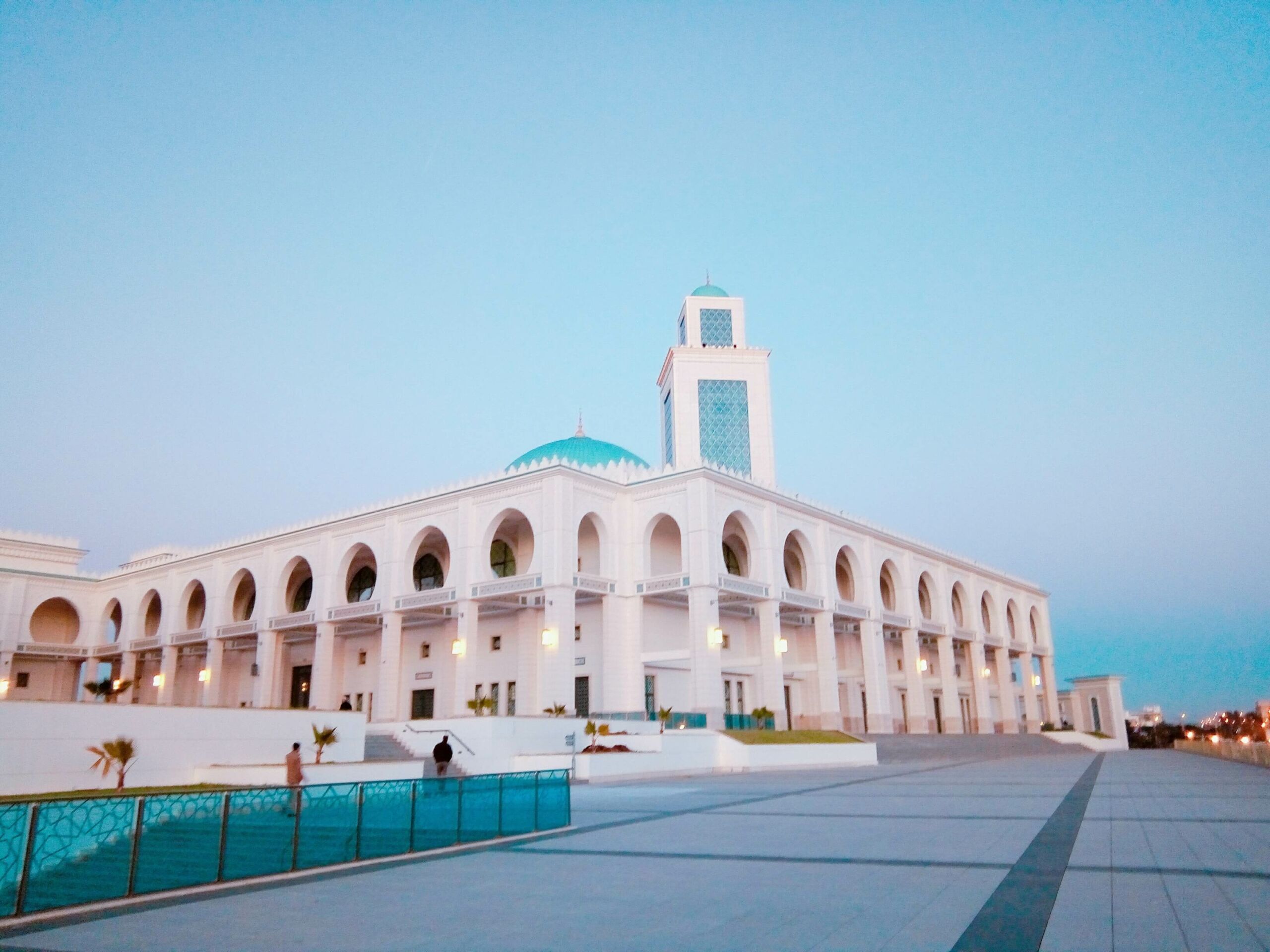
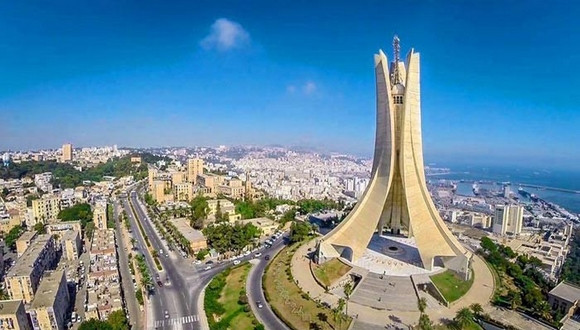
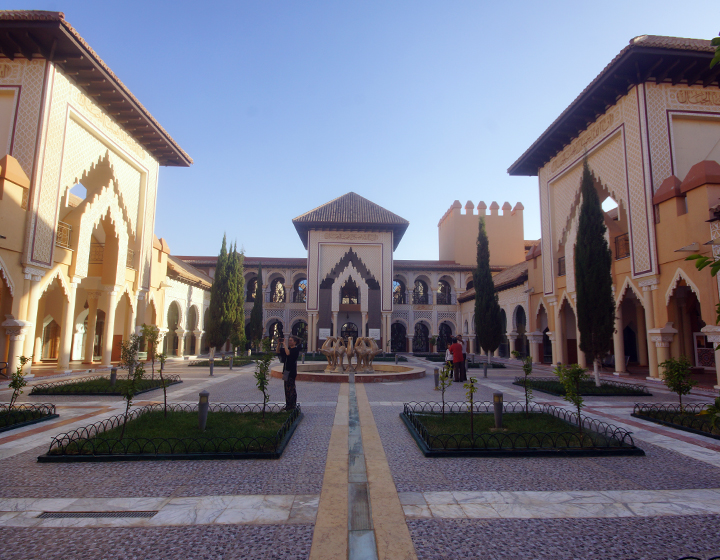
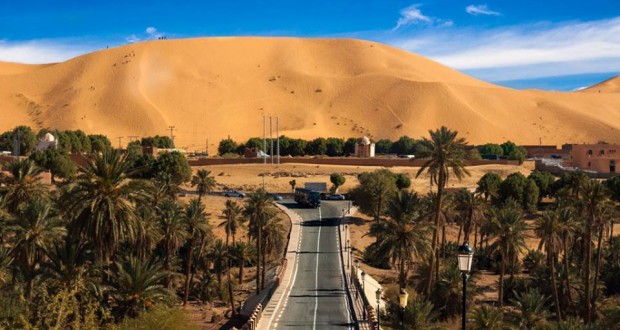
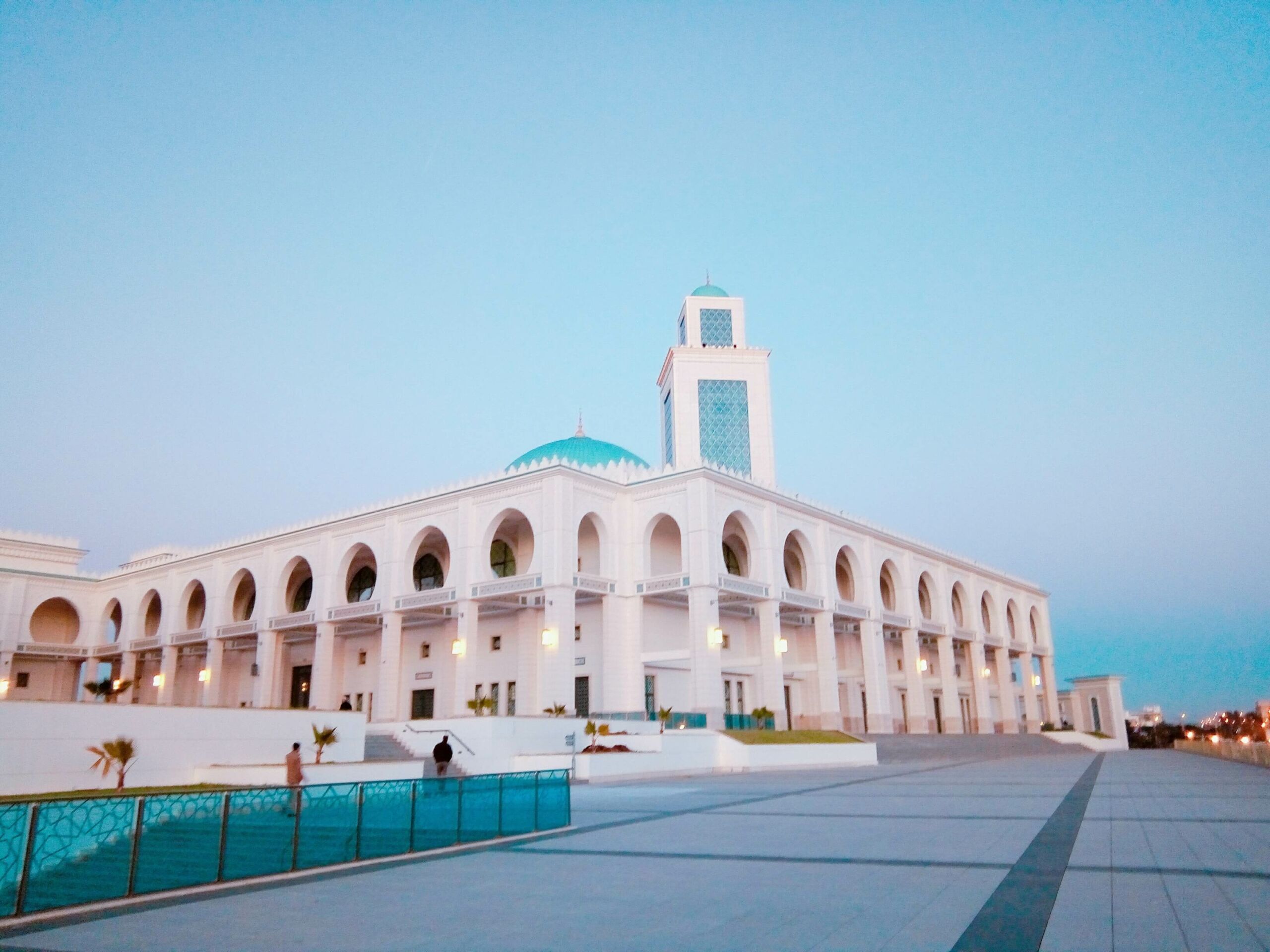
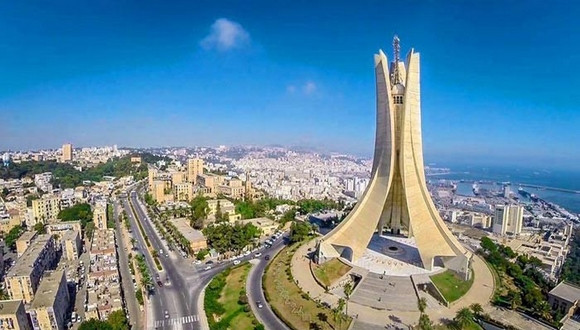
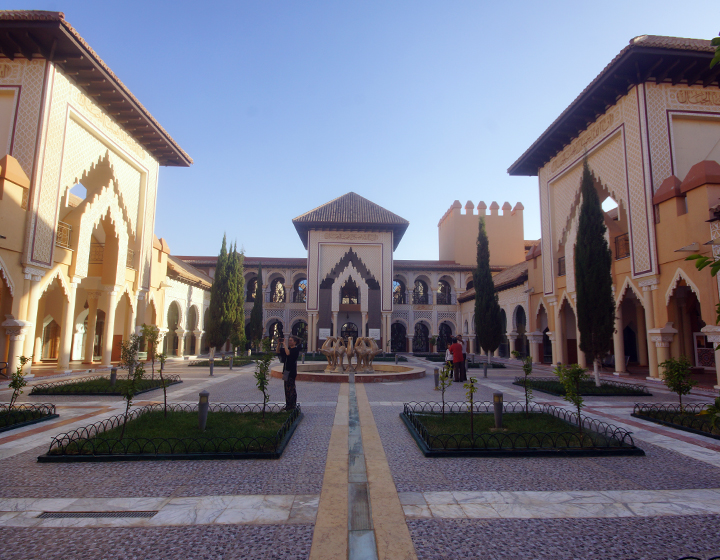
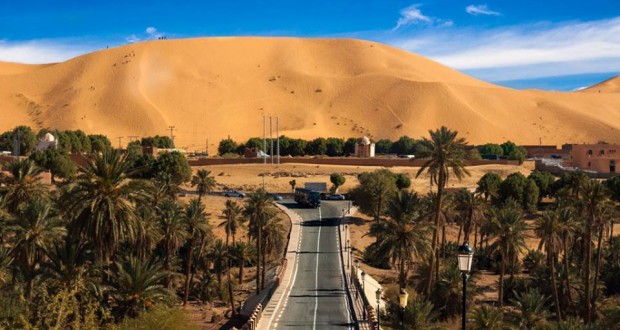
flex-wrap: wrap-reverse
justify-content
Controls how items are positioned along the main axis.
HTML
<div class="Flex_Container">
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
.Flex_Container {
display: flex;
justify-content: flex-start;
}
Result
justify-content: flex-start
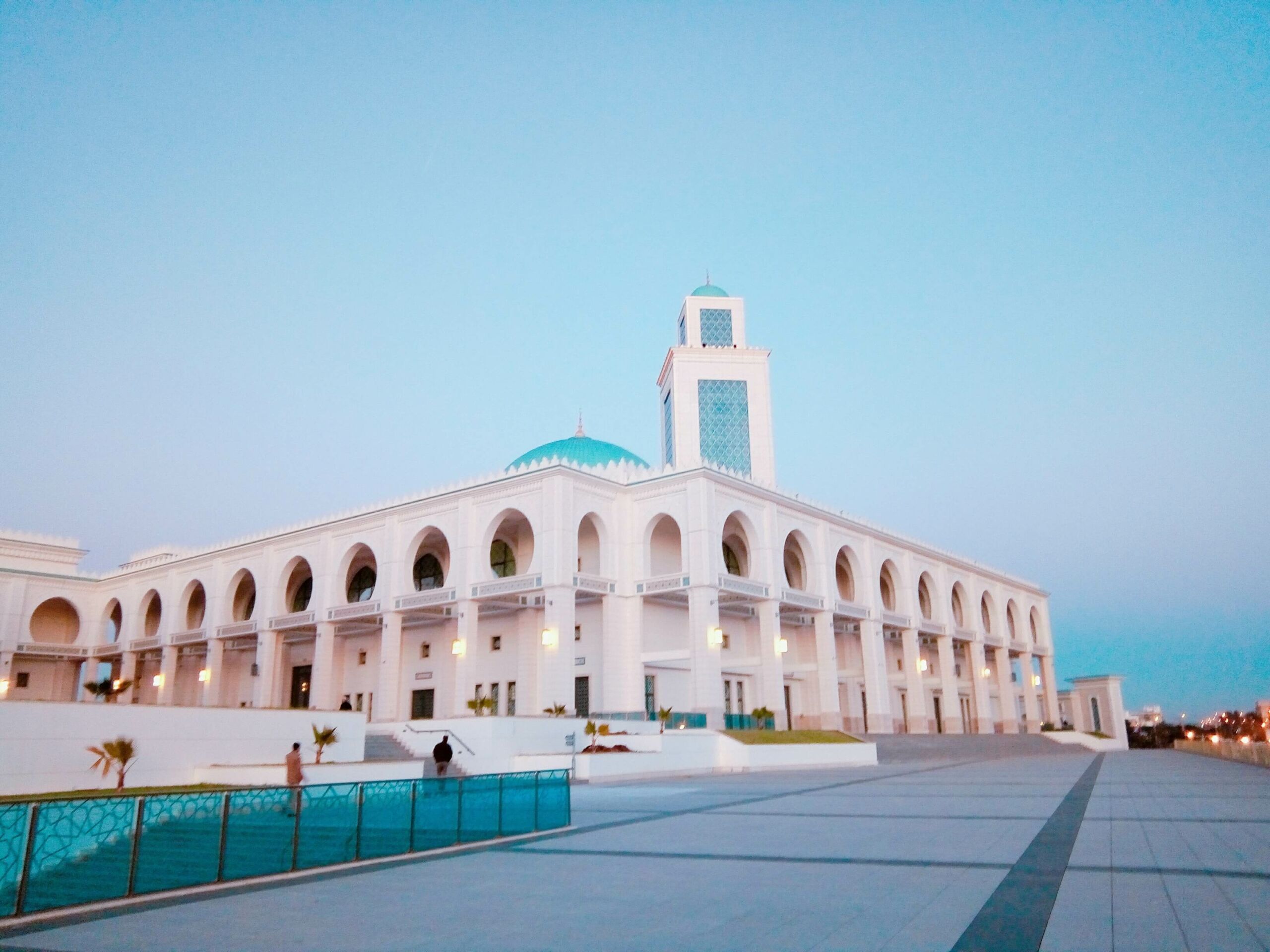
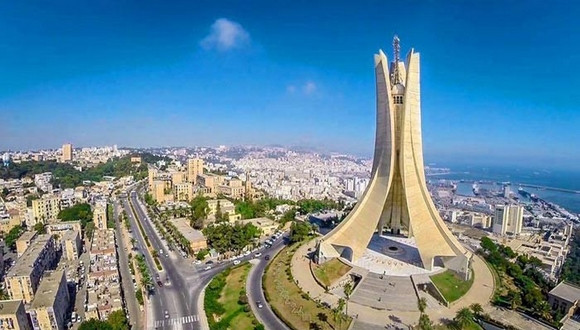
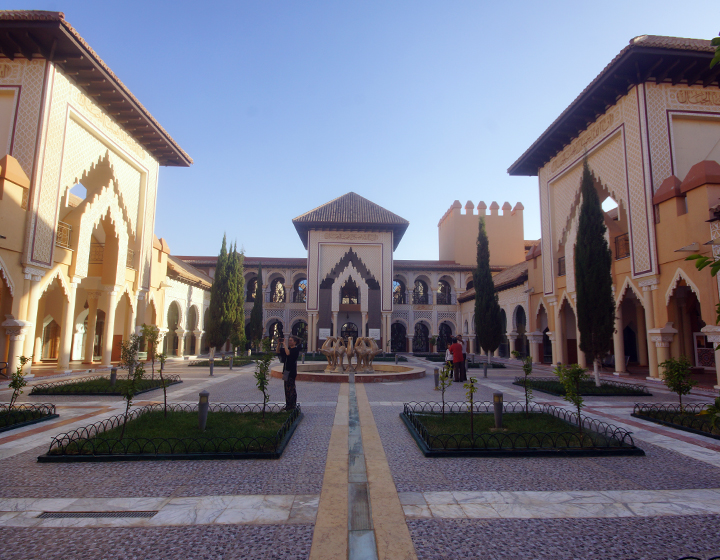
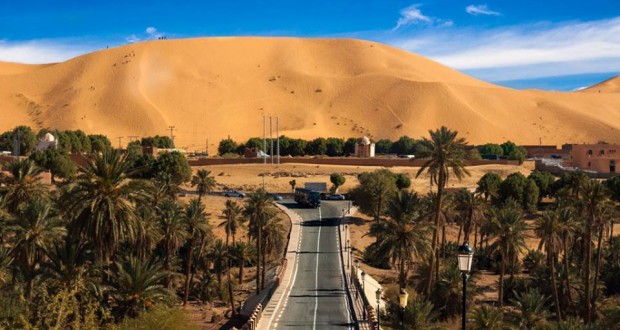
justify-content: flex-end
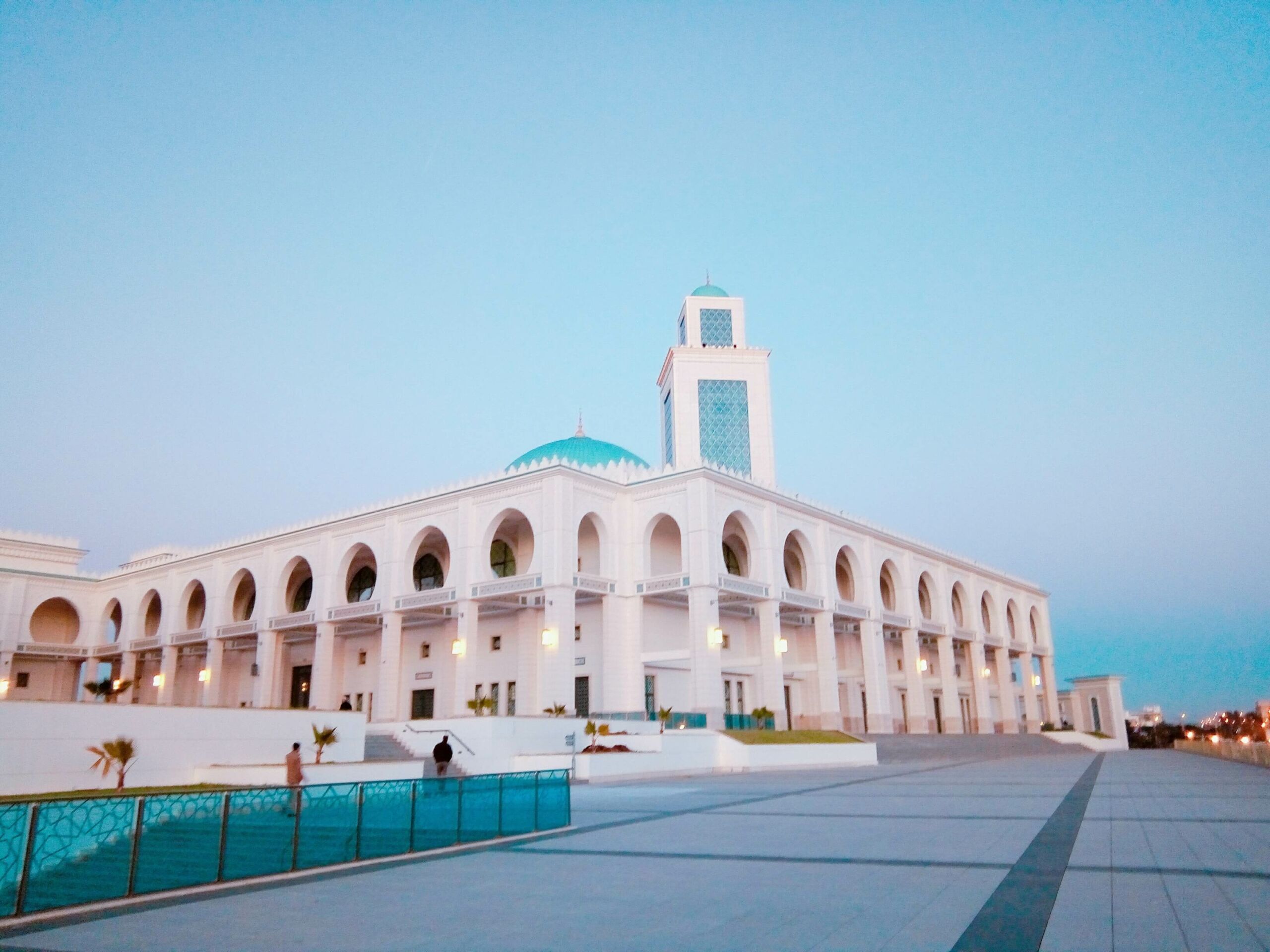
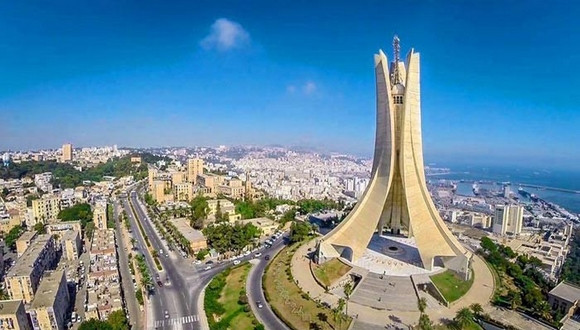
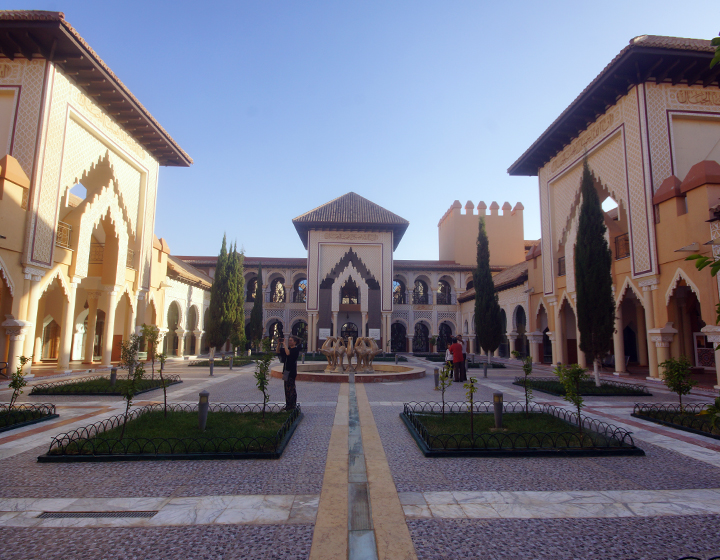
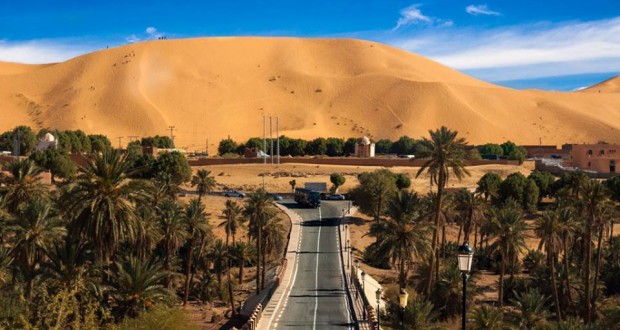
justify-content: center
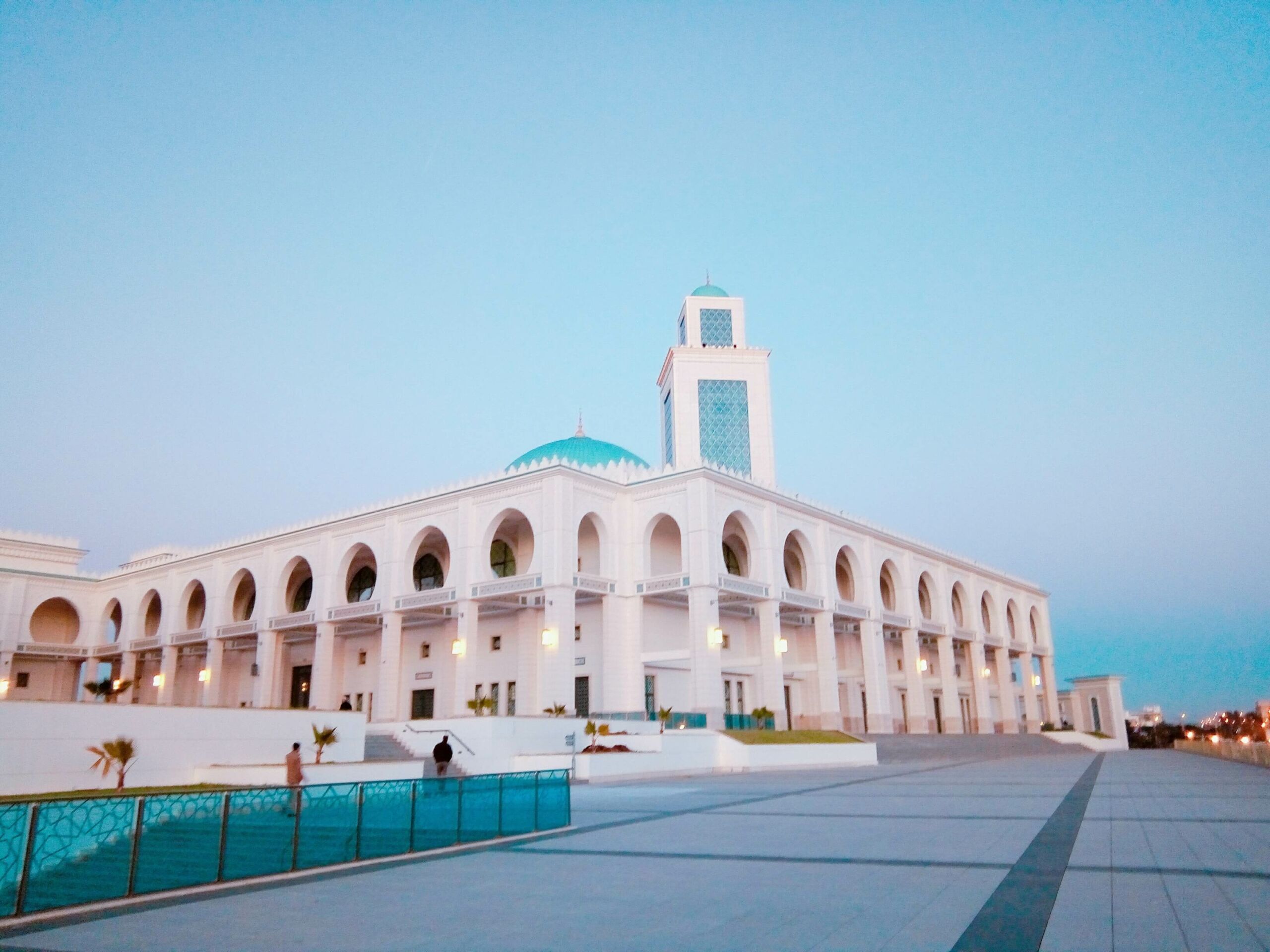
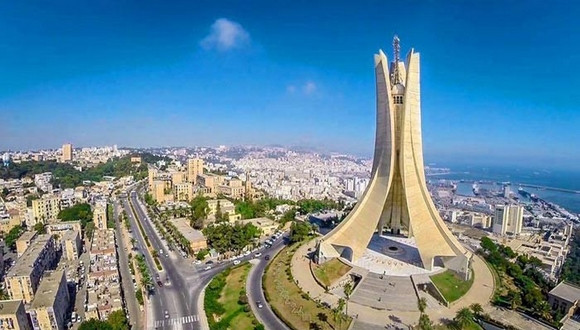
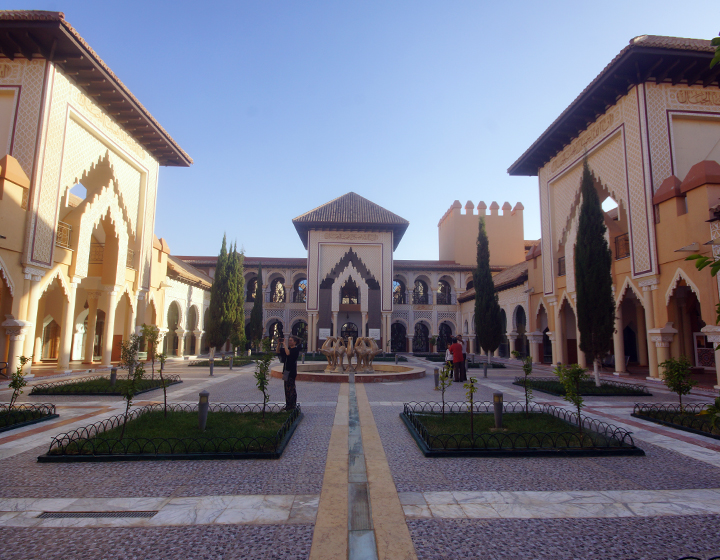
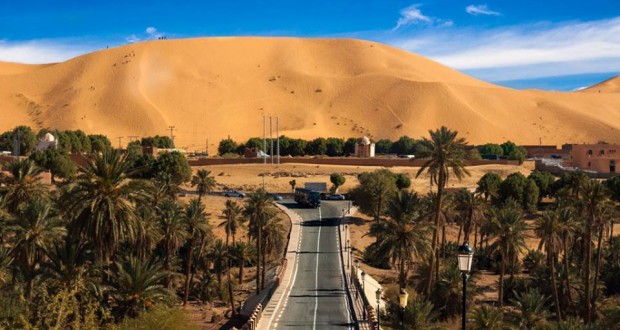
justify-content: space-between
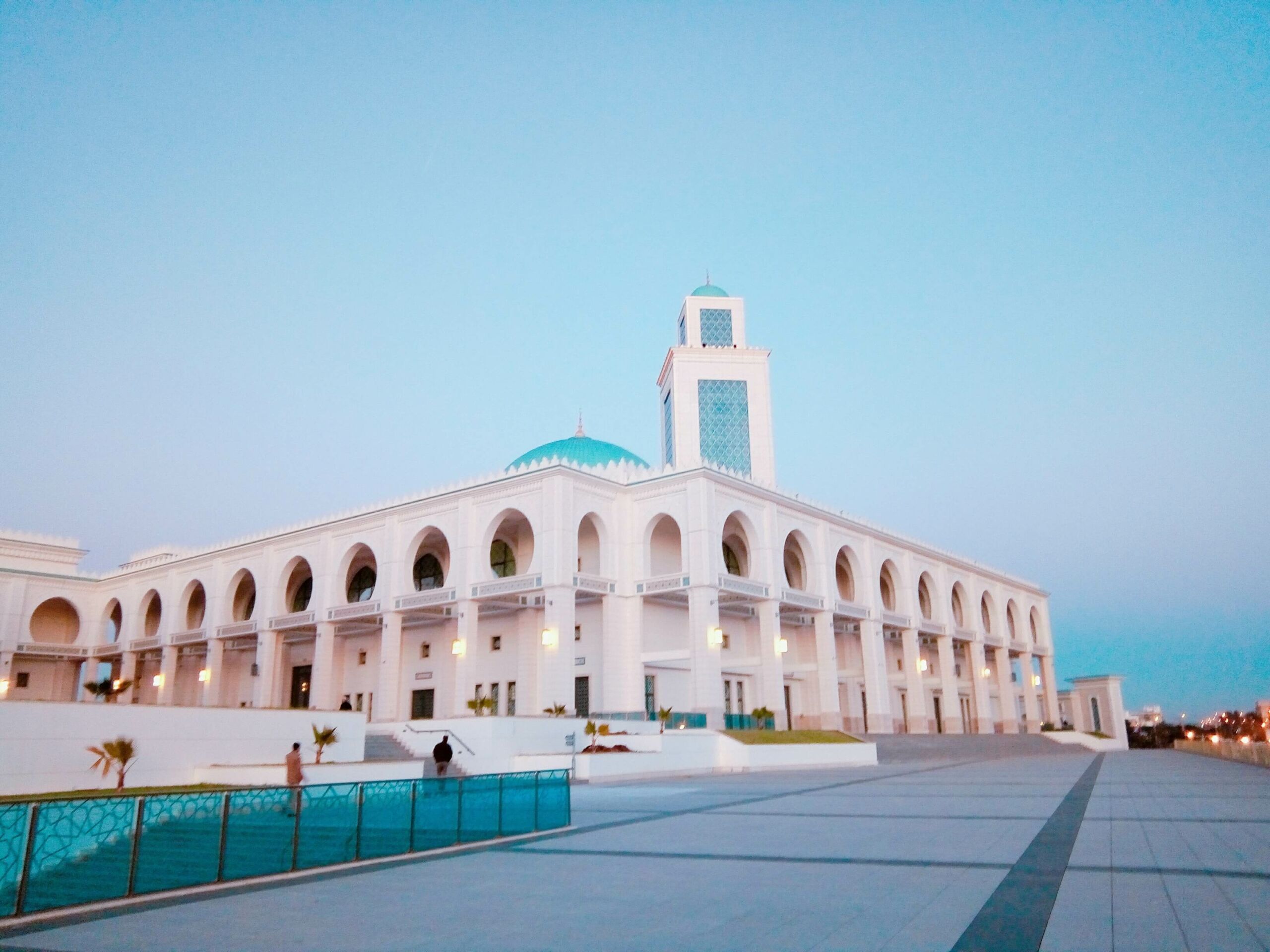
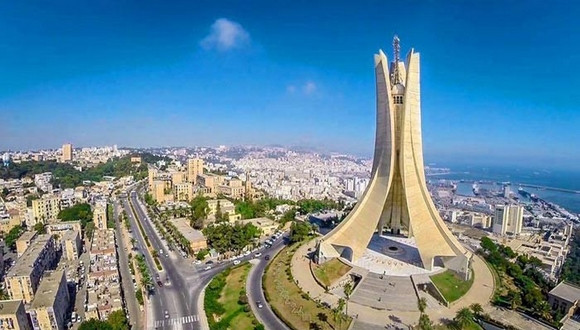
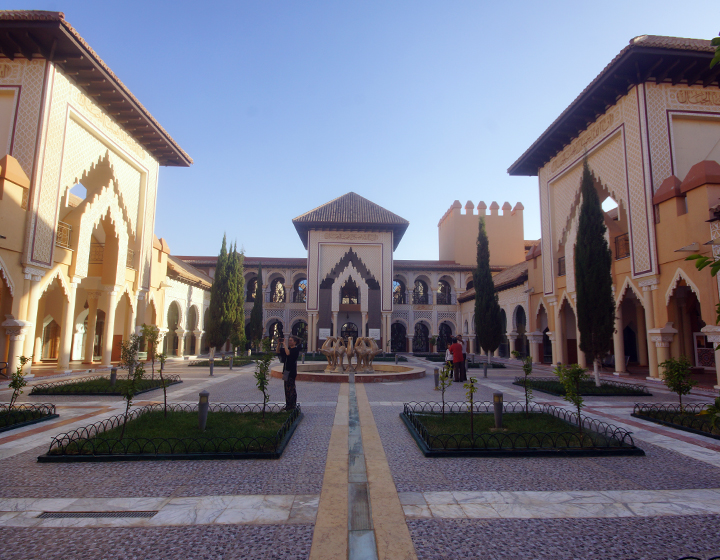
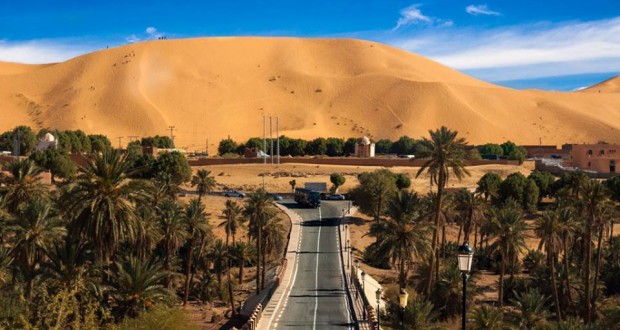
justify-content: space-around
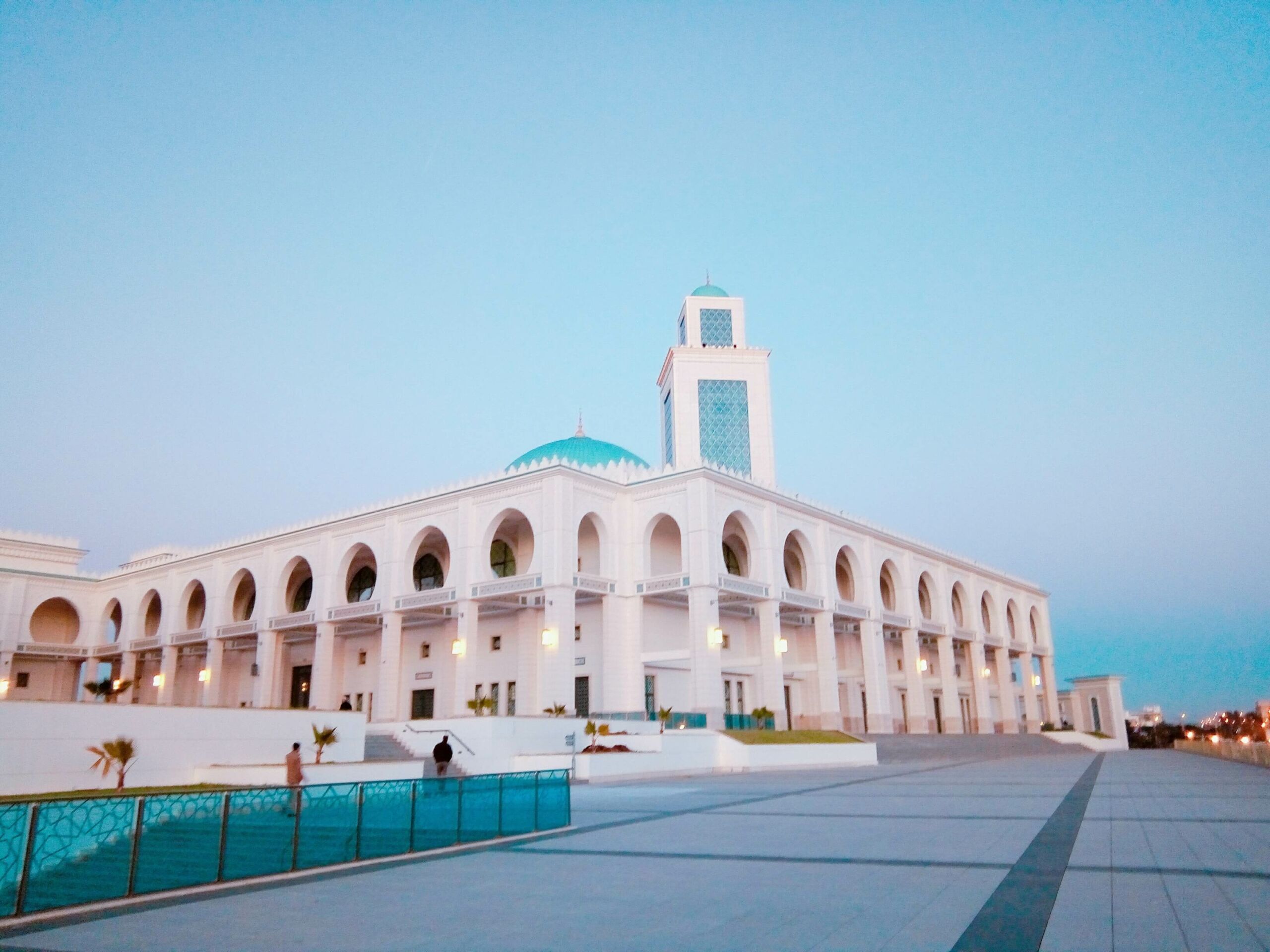
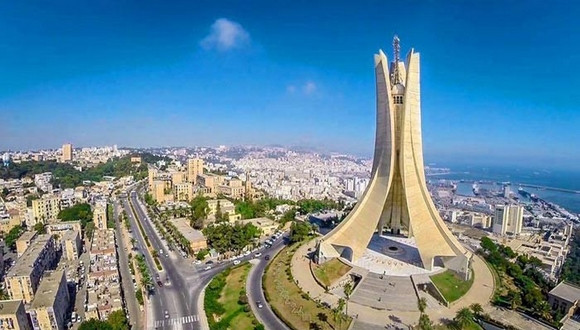
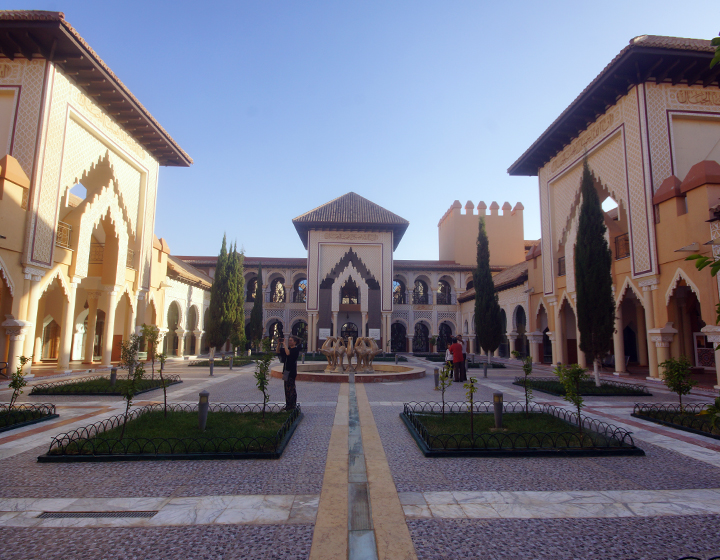
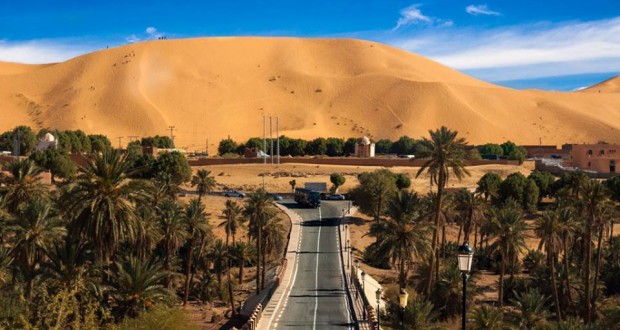
justify-content: space-evenly
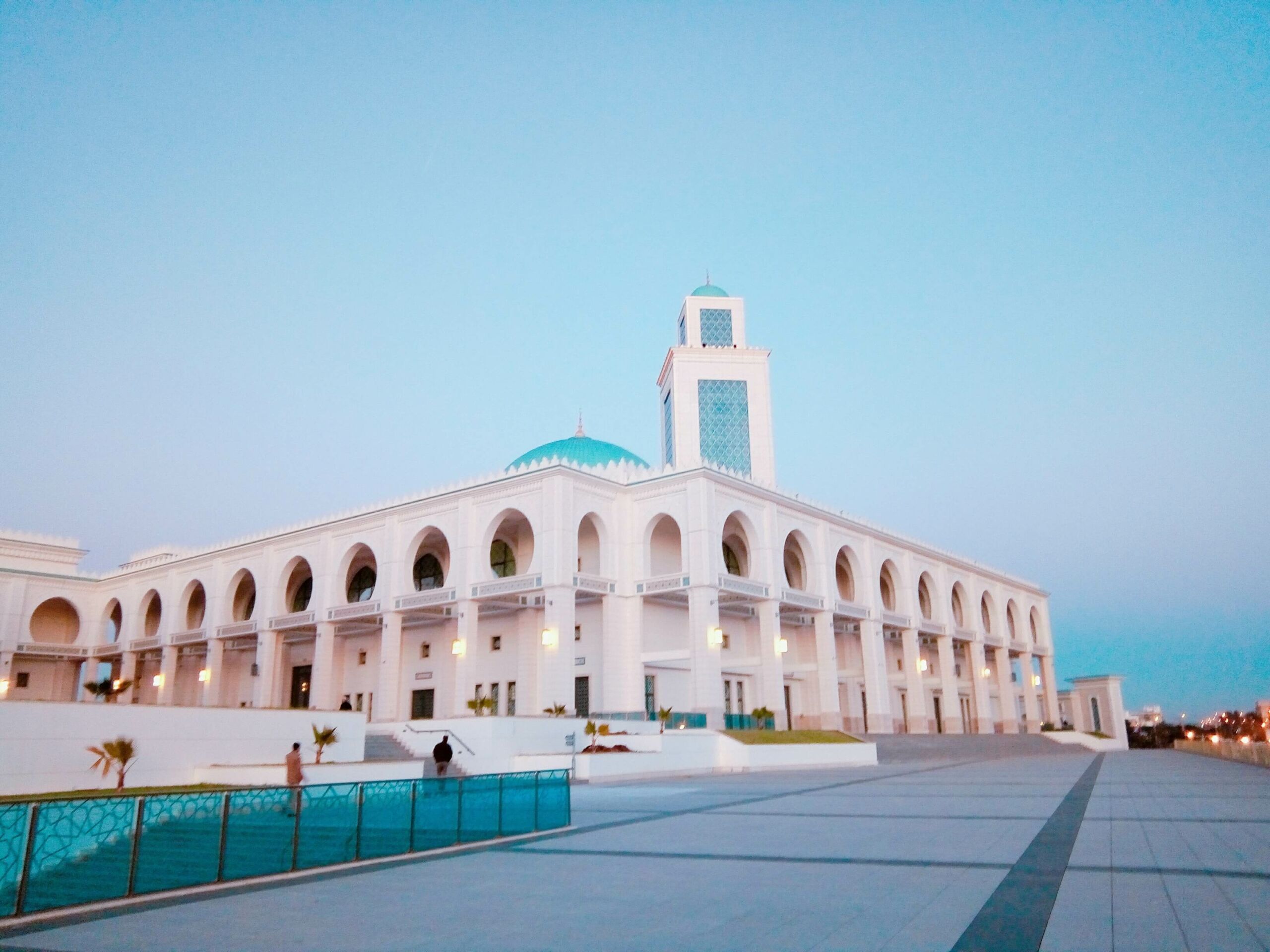
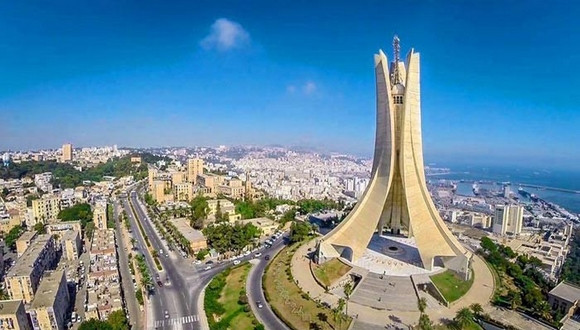
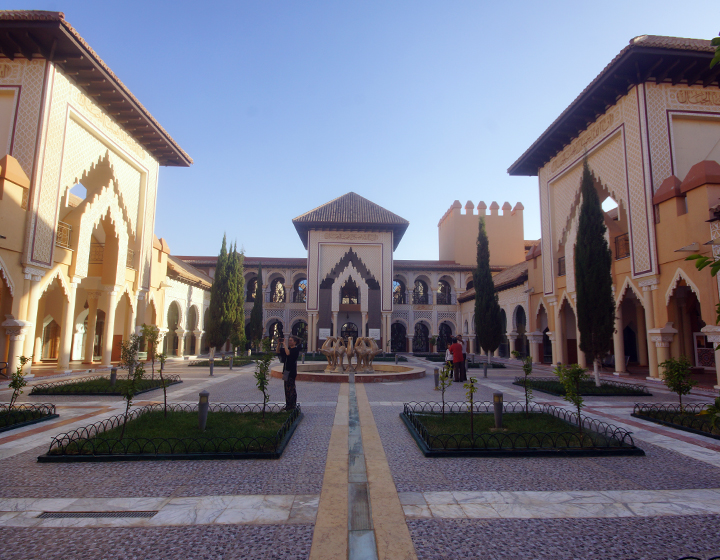
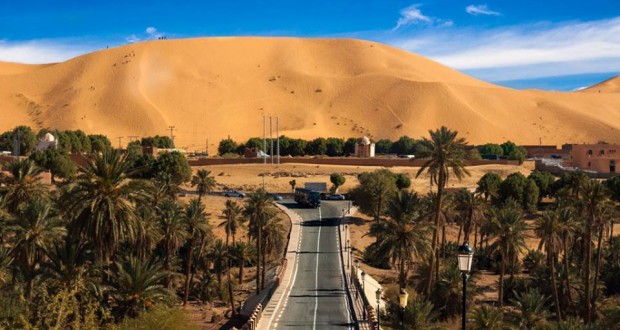
align-items
Controls how items are positioned along the cross axis.
HTML
<div class="Flex_Container">
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
.Flex_Container {
display: flex;
align-items: flex-start;
}
Result
align-items: flex-start
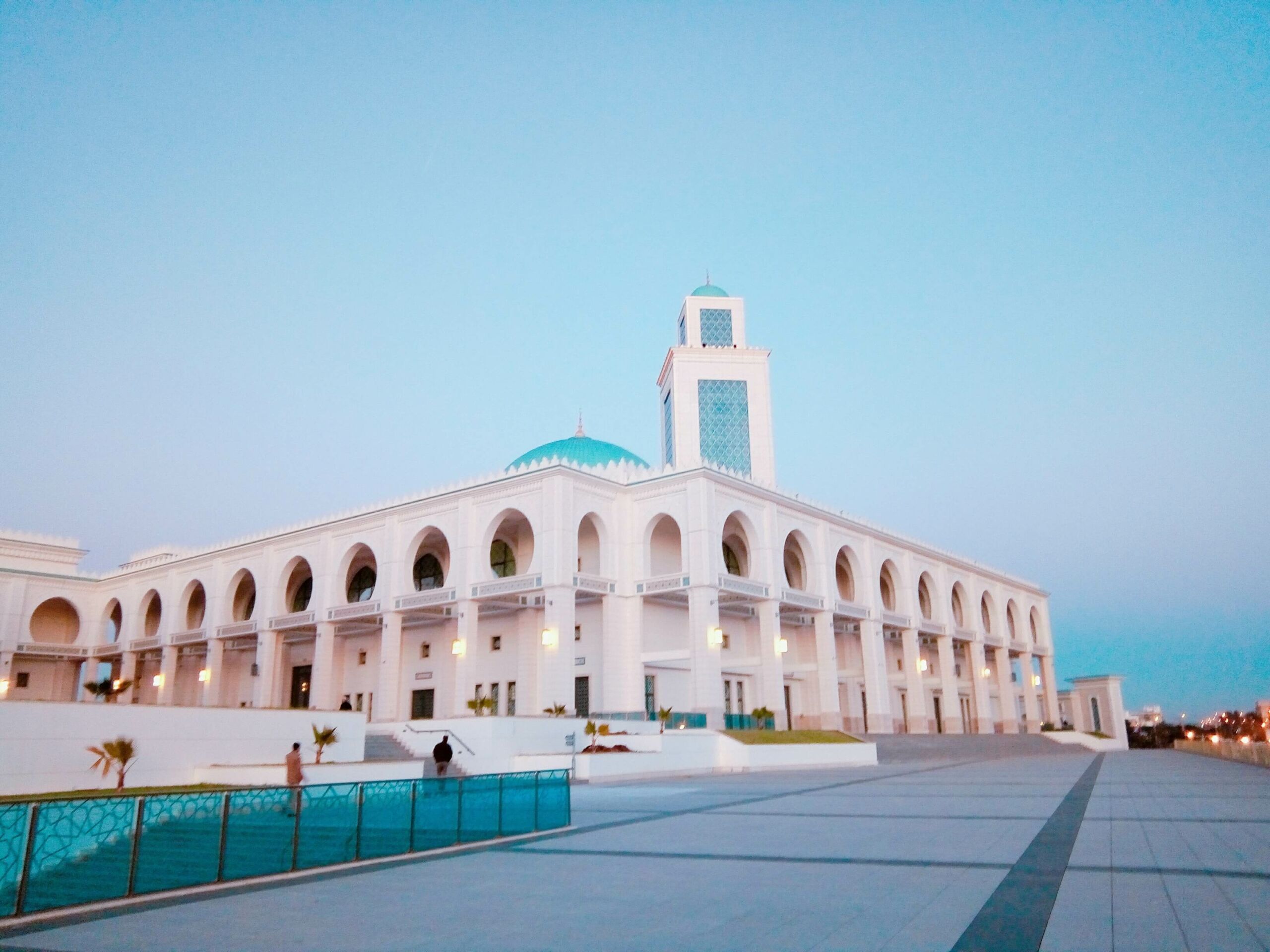
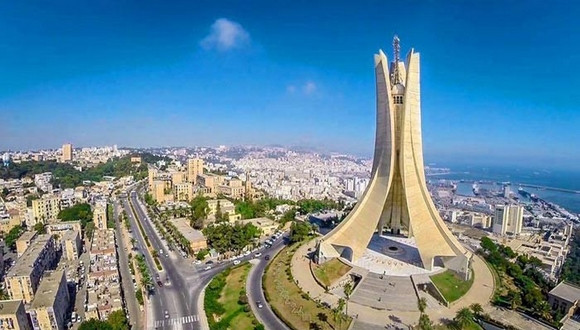
align-items: flex-end
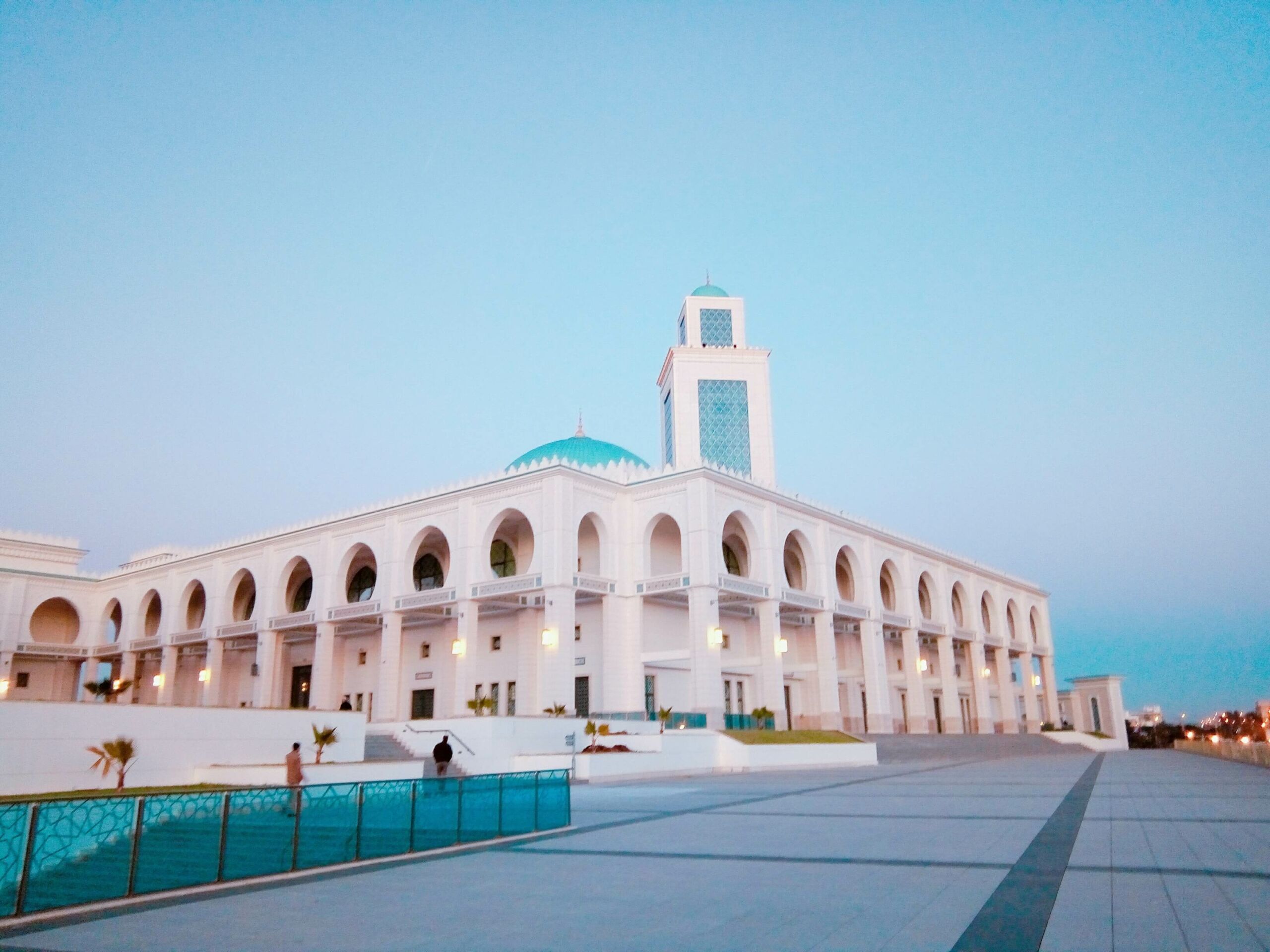
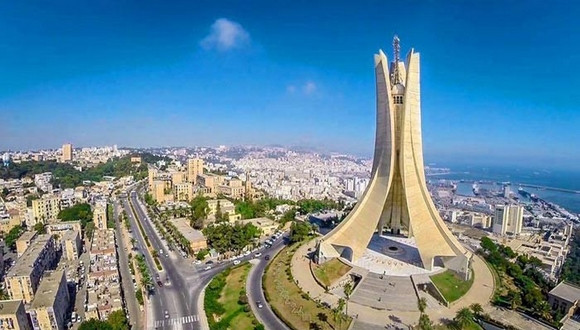
align-items: center
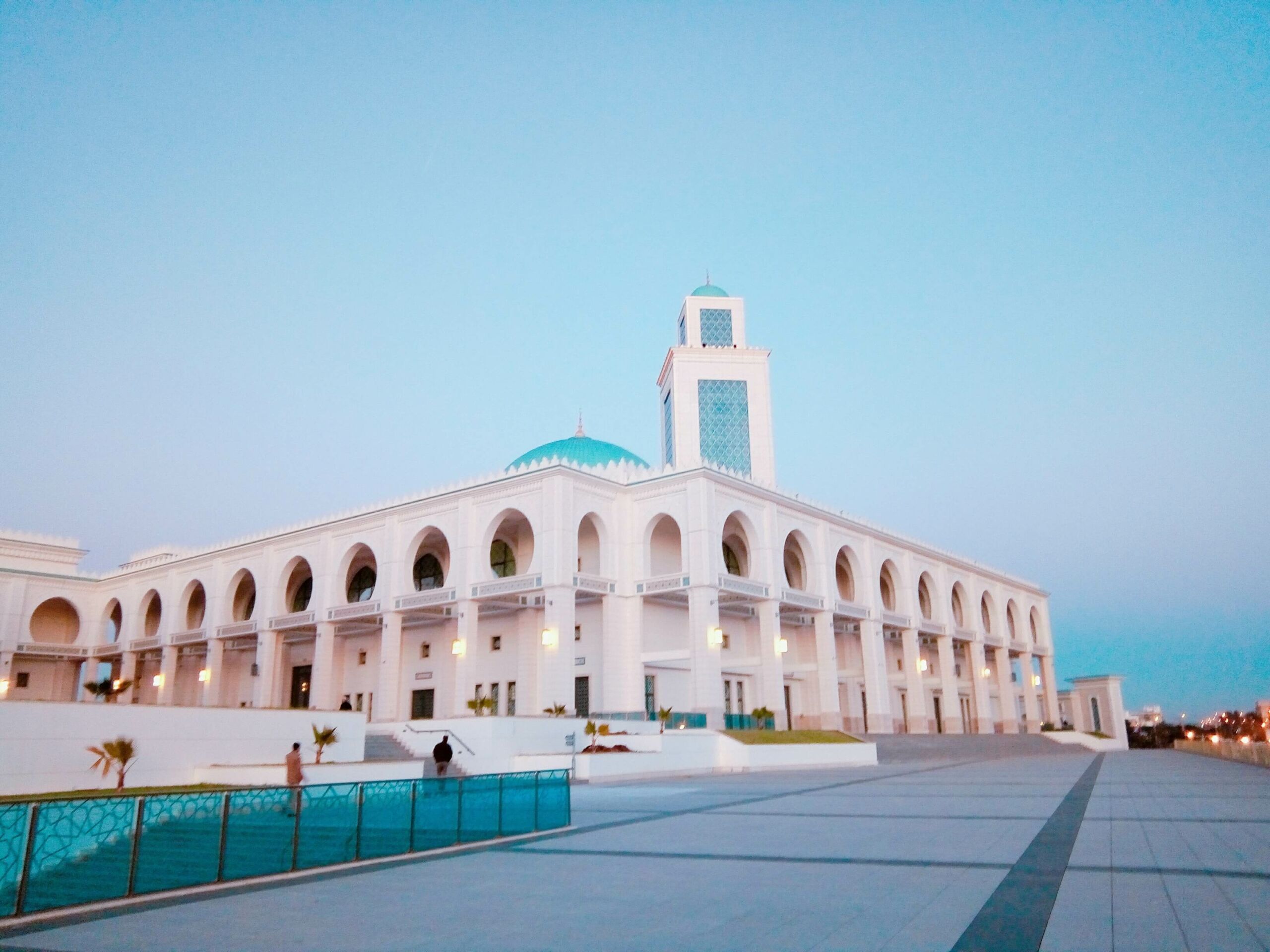
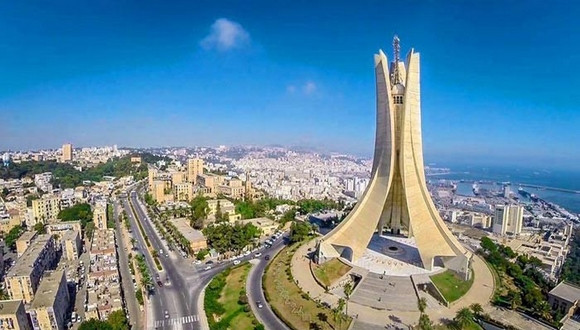
align-items: stretch
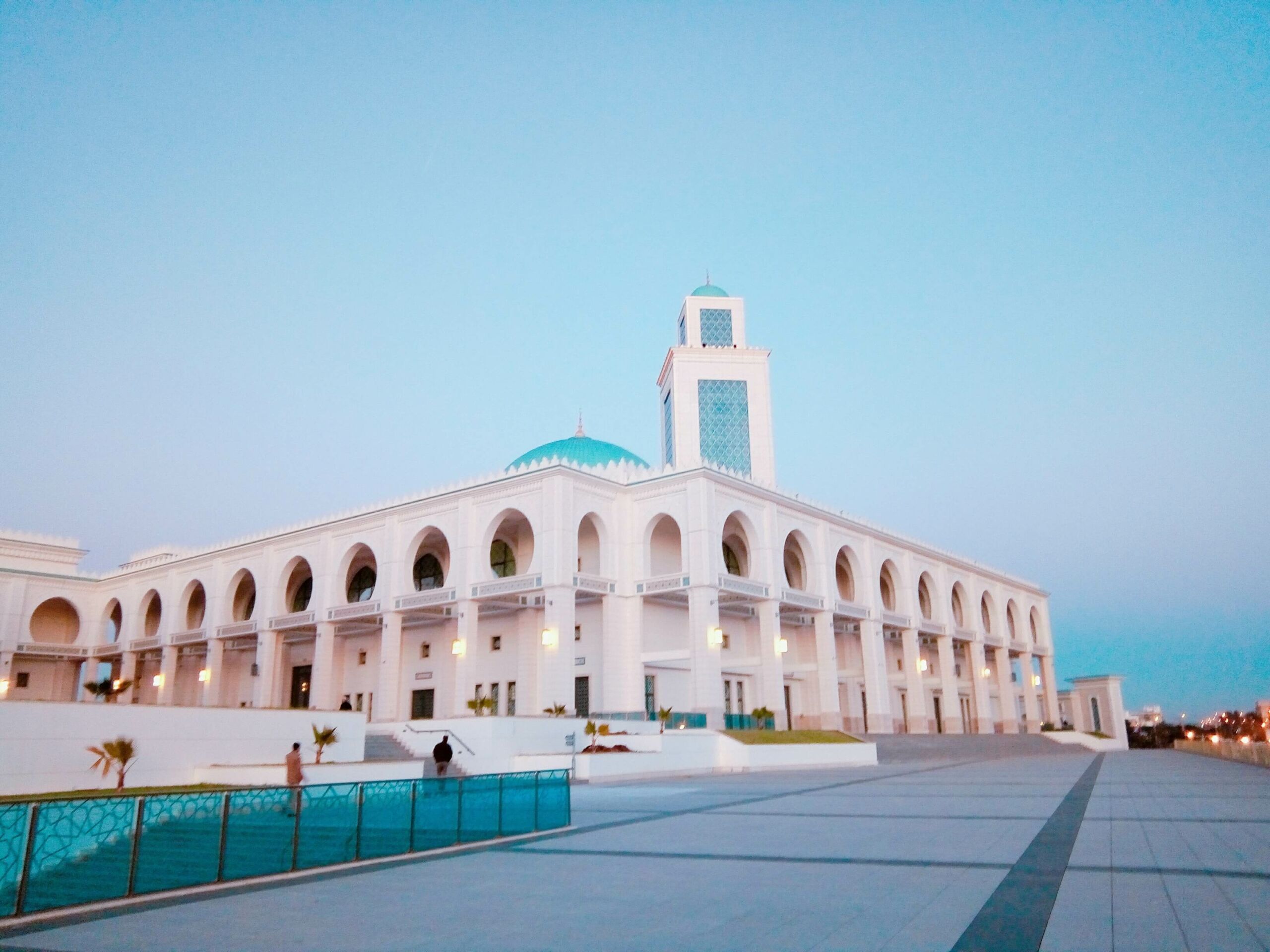
align-items: baseline
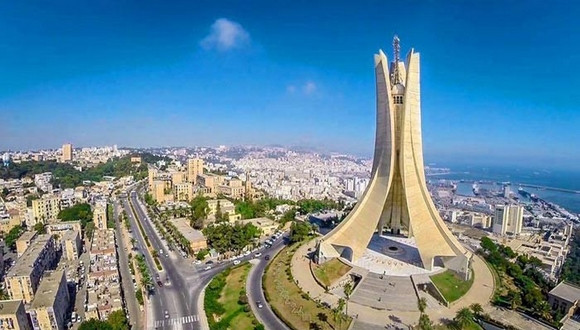
align-content
If flex-wrap is enabled, this controls the spacing of the flex rows along the cross axis. If items don't wrap, this property is ignored.
HTML
<div class="Flex_Container">
<div> </div>
<div> </div>
<div> </div>
<div> </div>
</div>
CSS
.Flex_Container {
display: flex;
align-content: flex-start;
}
Result
align-content: flex-start
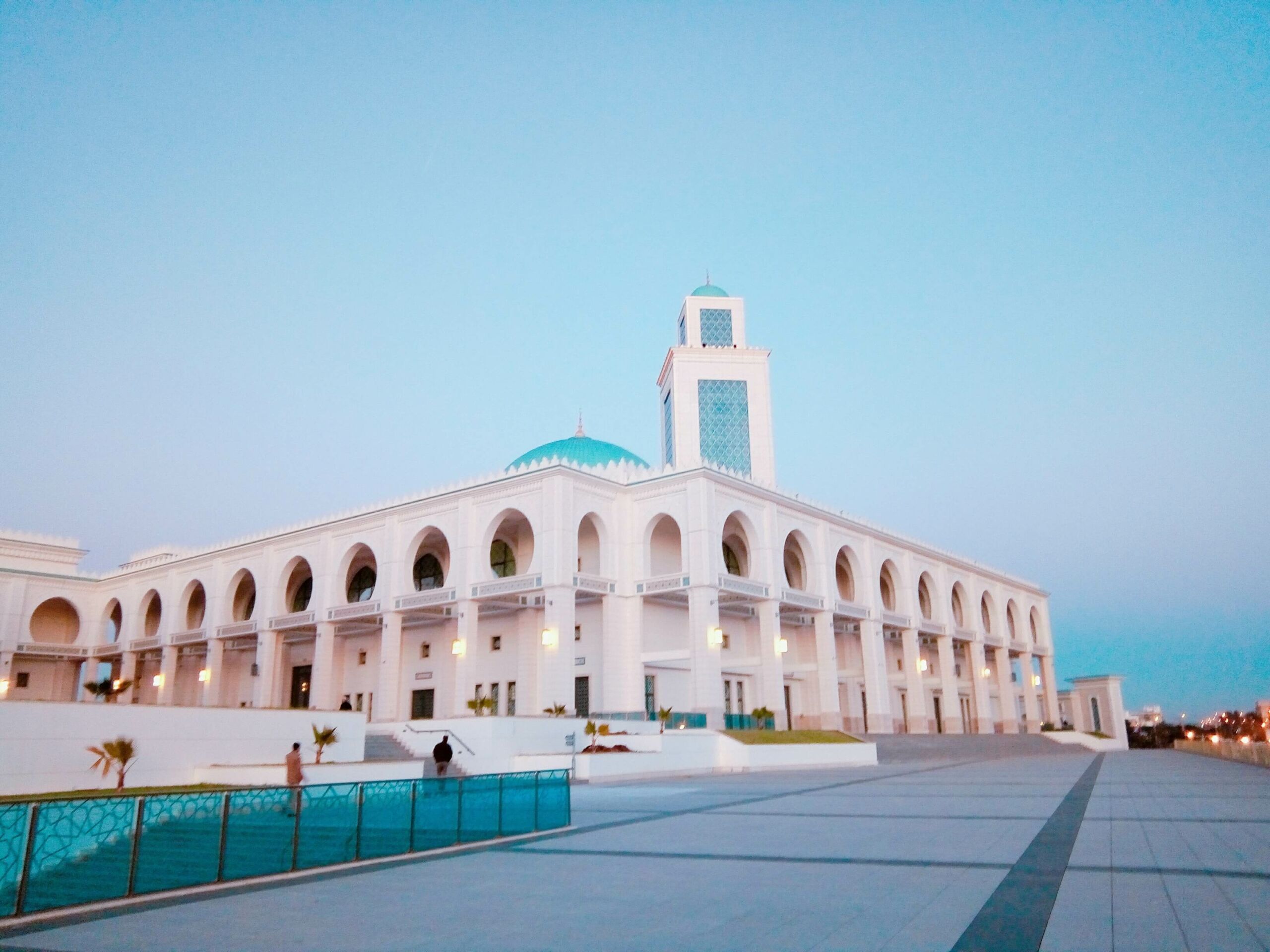
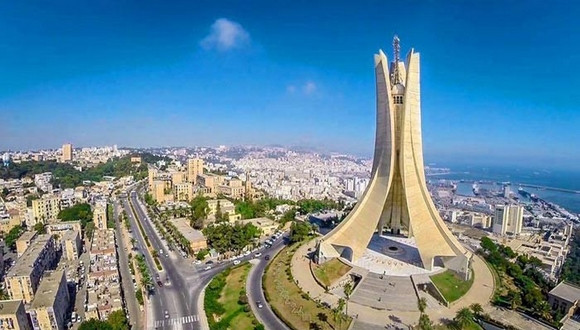
align-content: flex-end
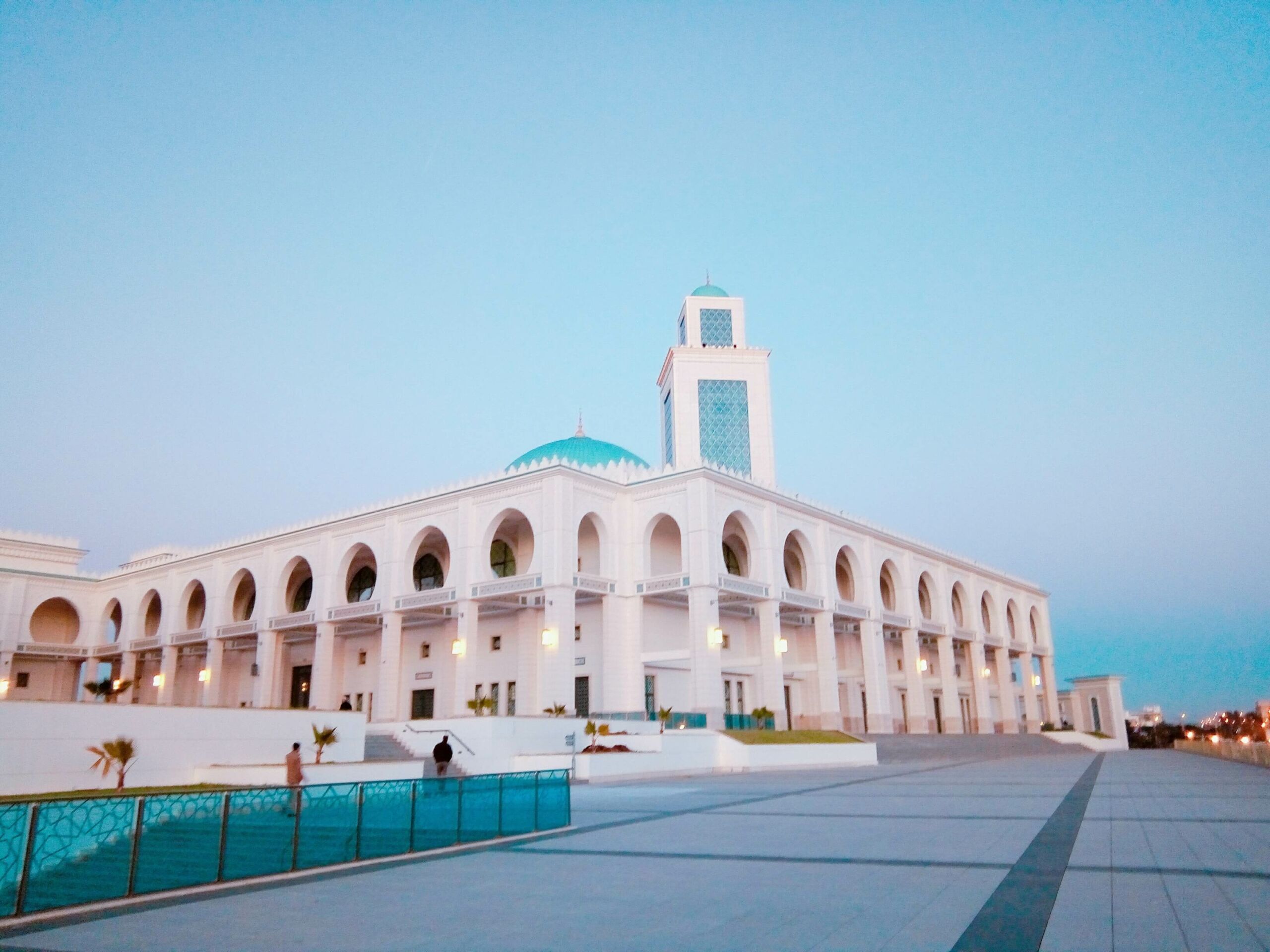
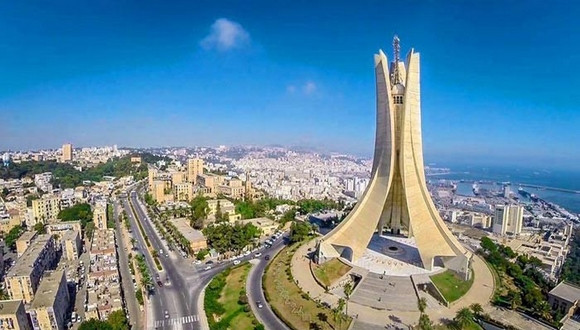
align-content: center
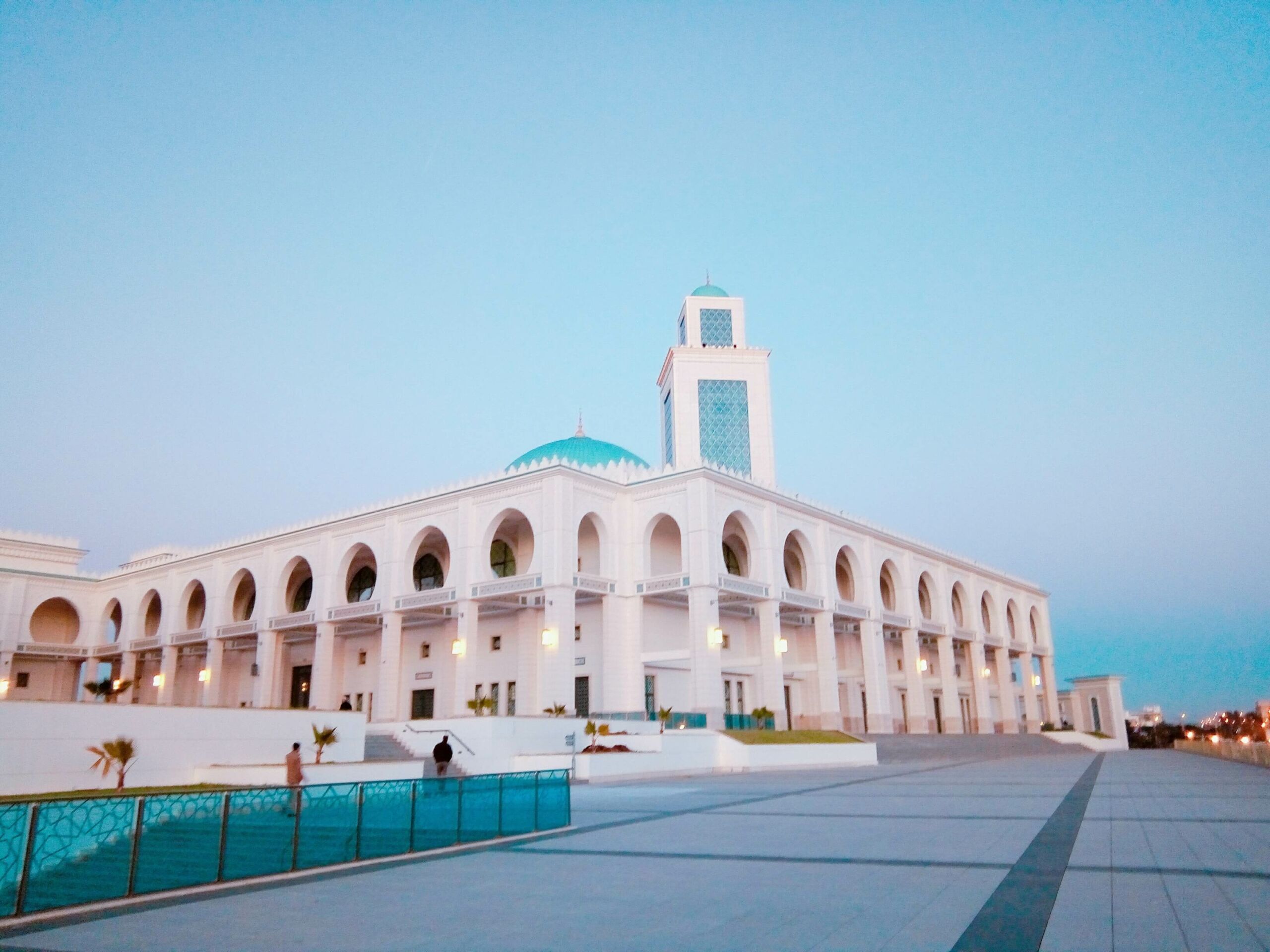
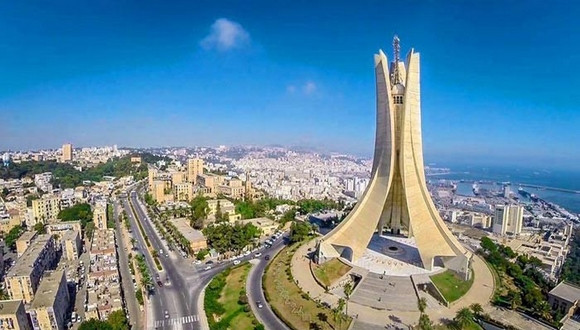
align-content: stretch
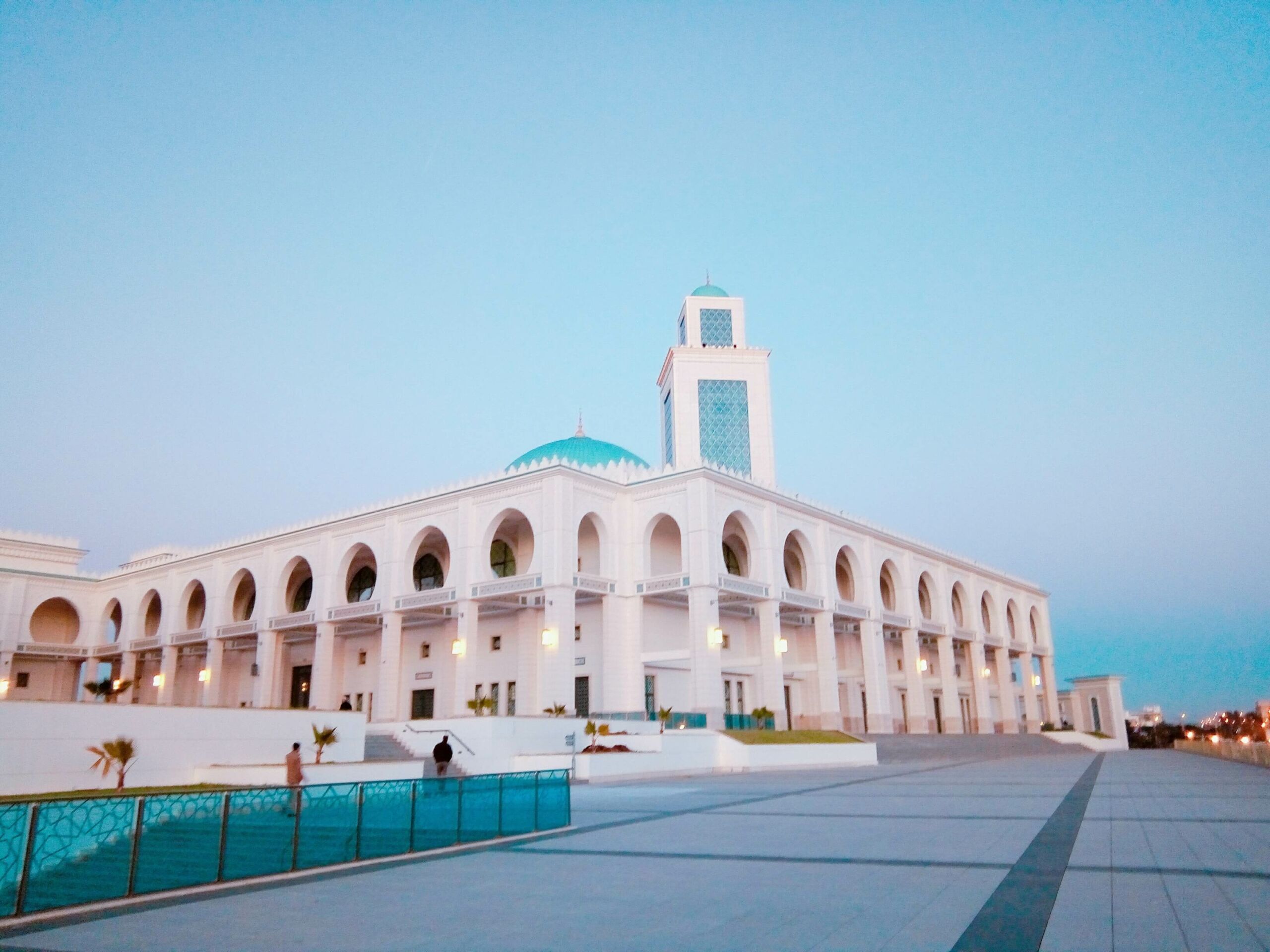
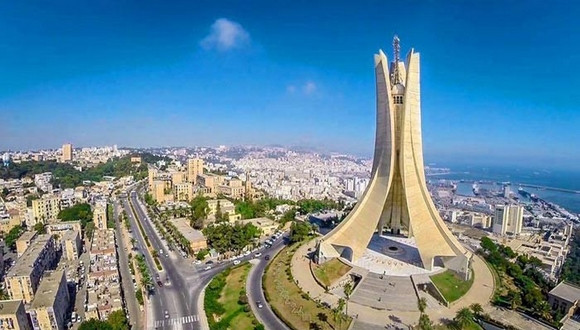
Order
order An integer that moves a flex item to a specific position among its siblings, disregarding source order. Initially, all flex items have an order of 0. Specifying a value of -1 to one item will move it to the beginning of the list, and a value of 1 will move it to the end. You can specify order values for each item to rearrange them however you wish. The numbers don't necessarily need to be consecutive.
HTML
<img class="Order_3" src="SRC/IMAGES/Tlemcen.jpg" alt="Tlemcen" / > <img class="Order_4" src="SRC/IMAGES/Oran.jpg" alt="Oran" / > <img class="Order_1" src="SRC/IMAGES/Algiers.jpg" alt="Algiers" / > <img class="Order_2" src="SRC/IMAGES/Constantine.jpg" alt="Constantine"/ >
CSS
.Order_1 {
order: 1;
}
Result
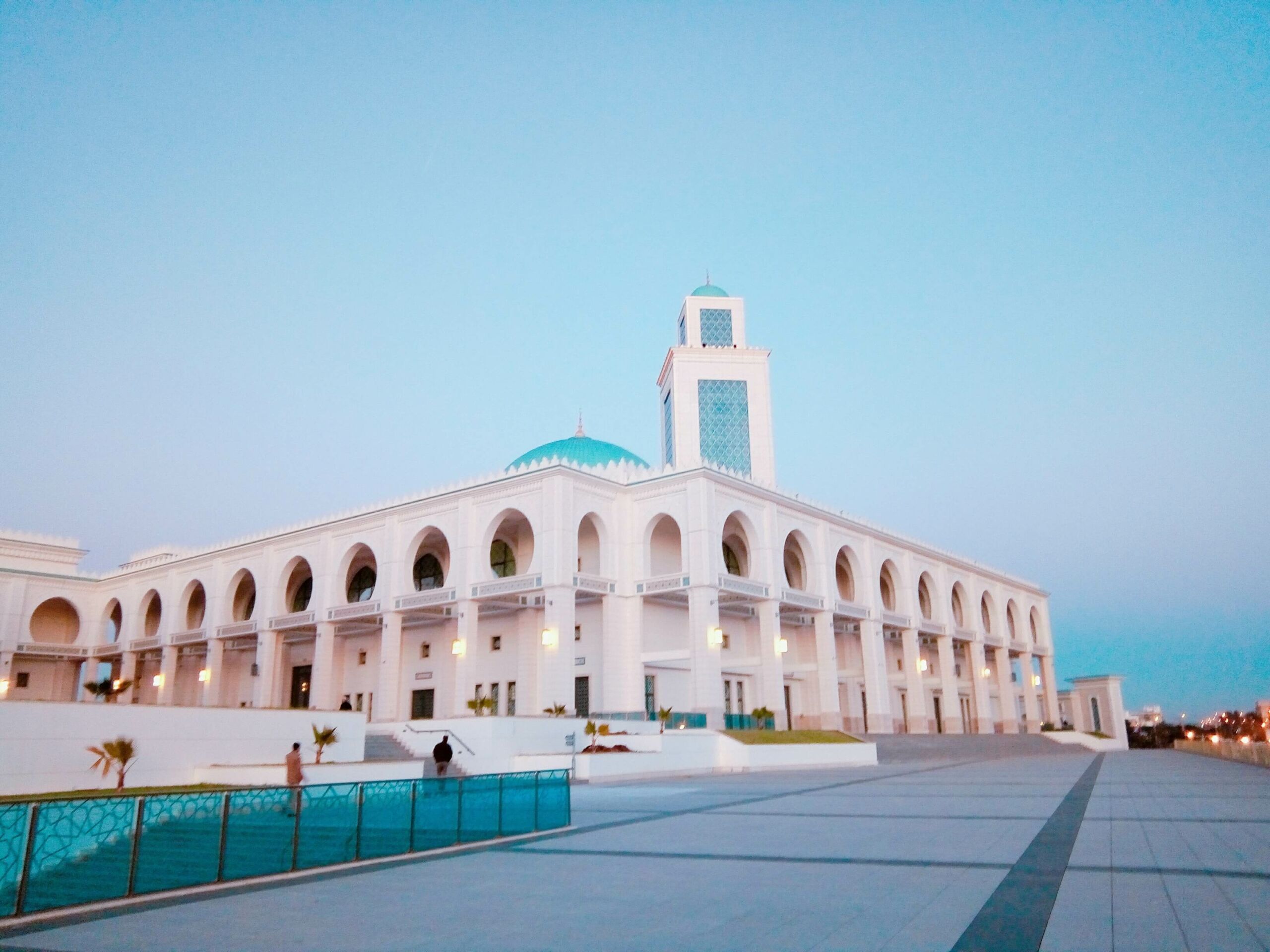
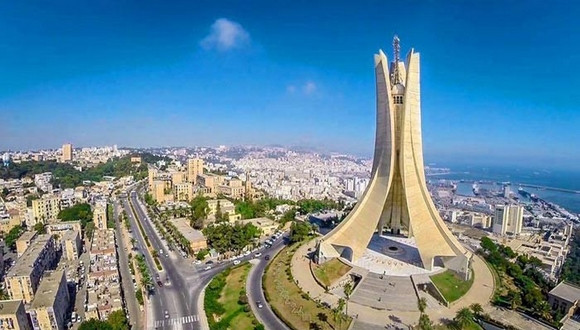
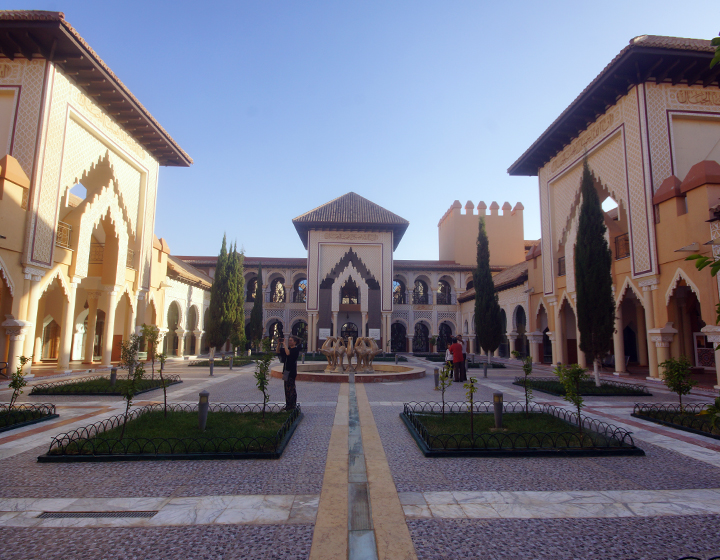
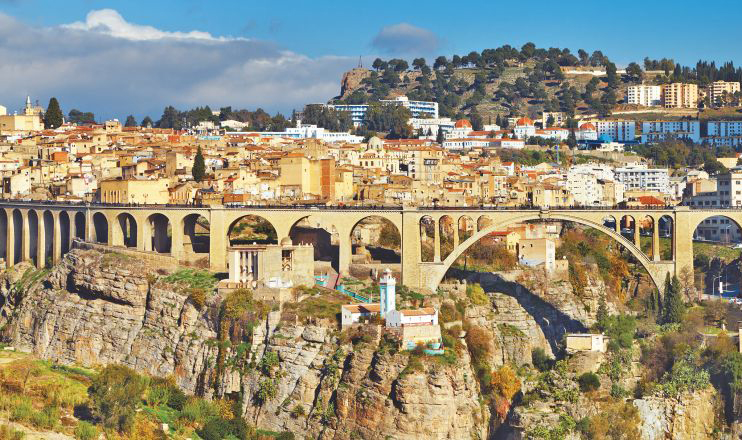
Grid
Grid lets you define a two-dimensional layout of columns and rows and then place elements within the grid. An element with display: grid becomes a grid container. Its child elements then become grid items. Use grid-template-columns and grid-template-rows to define the size of each of the columns and rows in the grid.
HTML
<div class="Grid" >
<div > </div >
<div > </div >
<div > </div >
</div >
CSS
.Grid {
display:grid;
grid-template-columns: 1fr 1fr 1fr;
grid-template-rows: 1fr 1fr;
}
Result
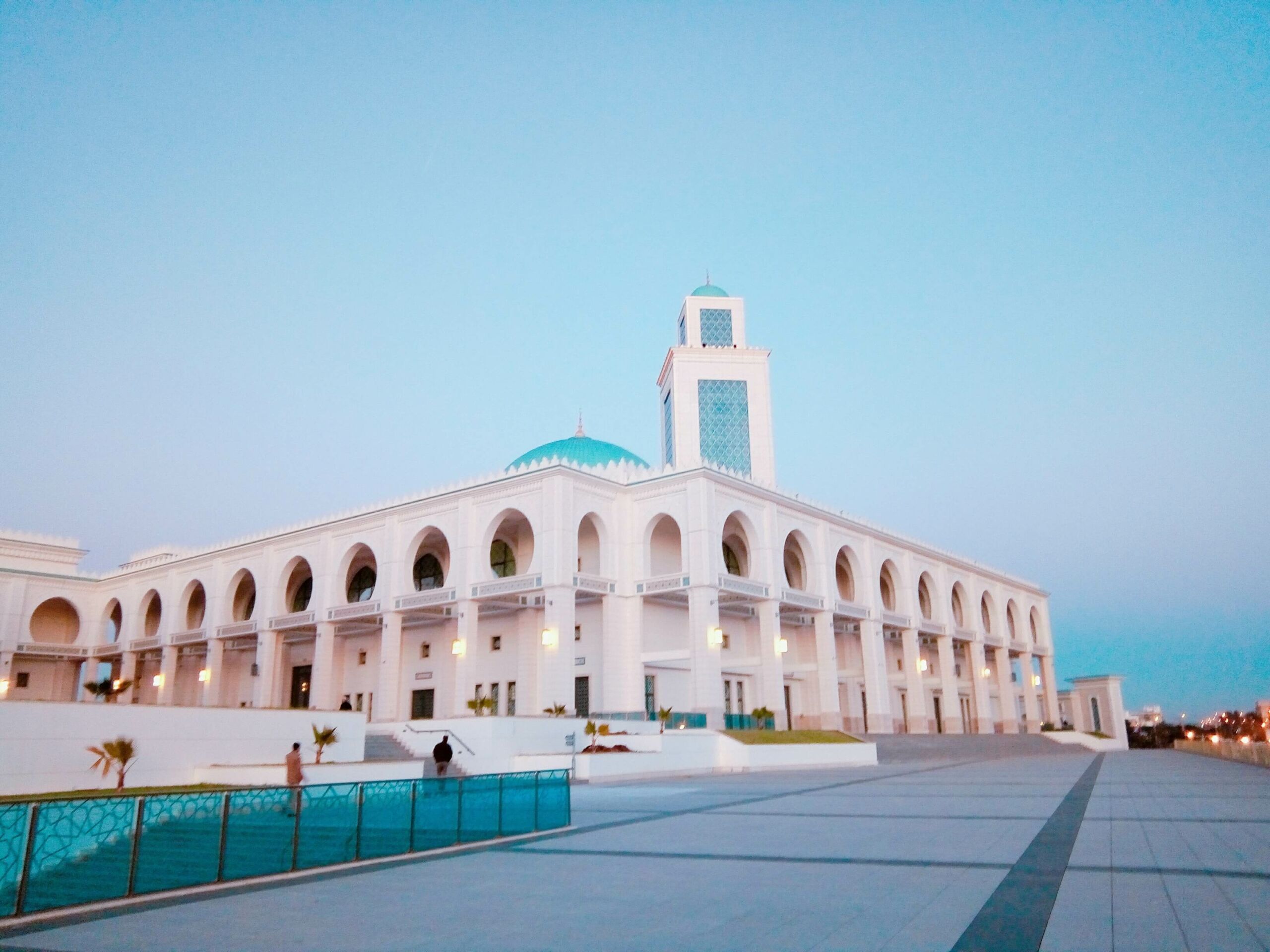
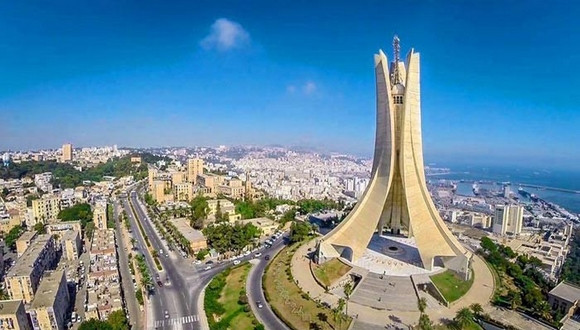
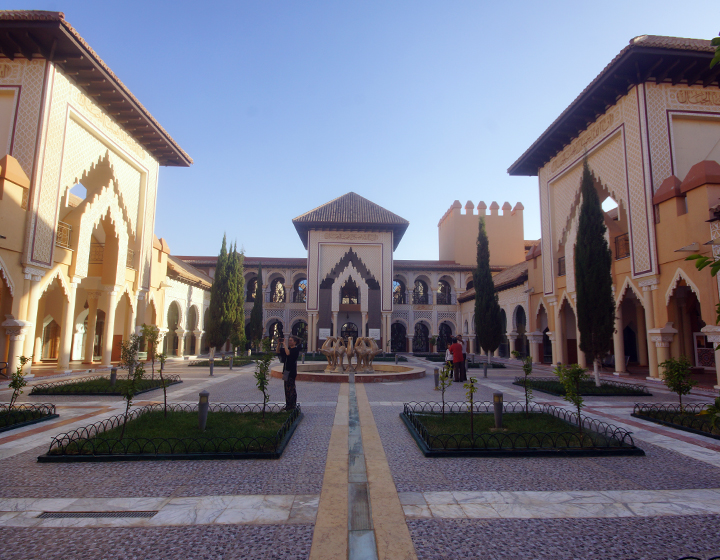
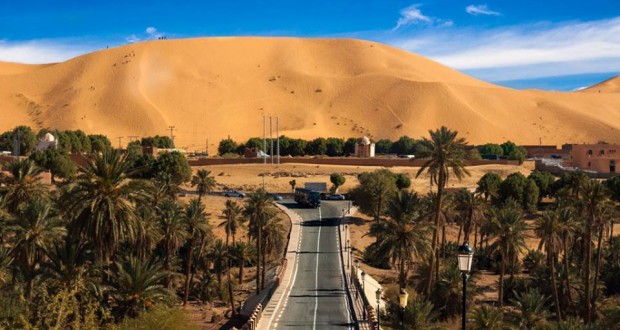
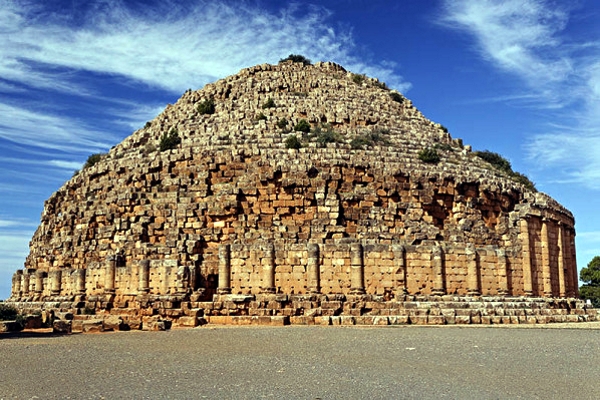
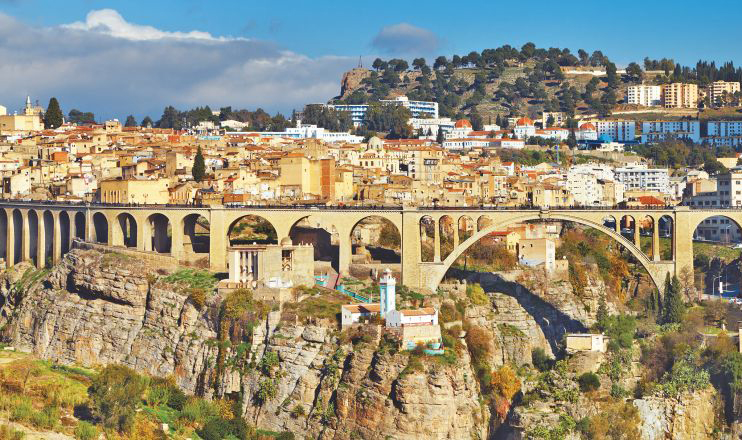
HTML
<div class="Grid" >
<div > </div >
<div > </div >
<div > </div >
</div >
CSS
.Grid {
display:grid;
grid-template-columns: 2fr 1fr;
grid-template-rows: 1fr 1fr;
}
Result
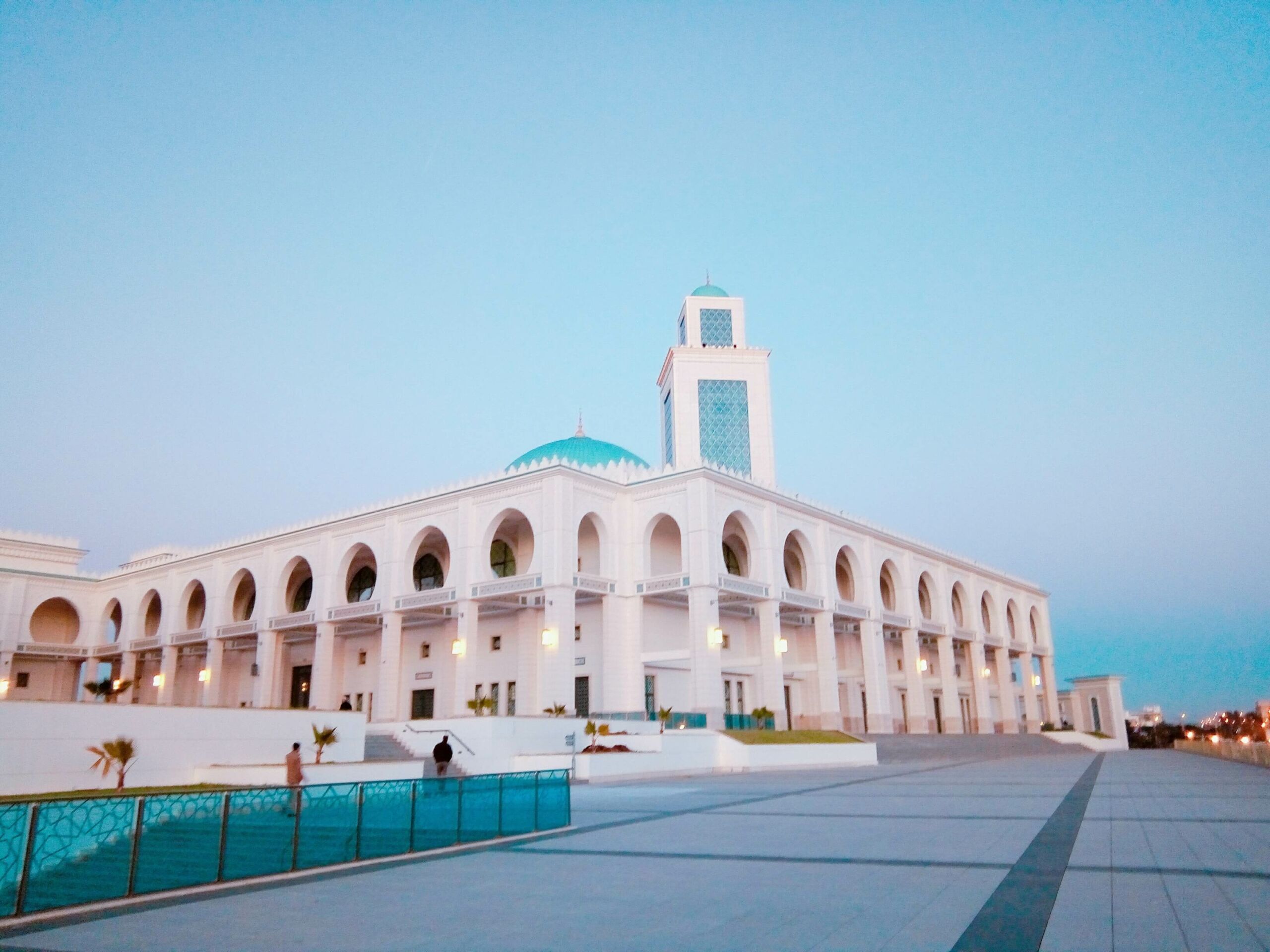
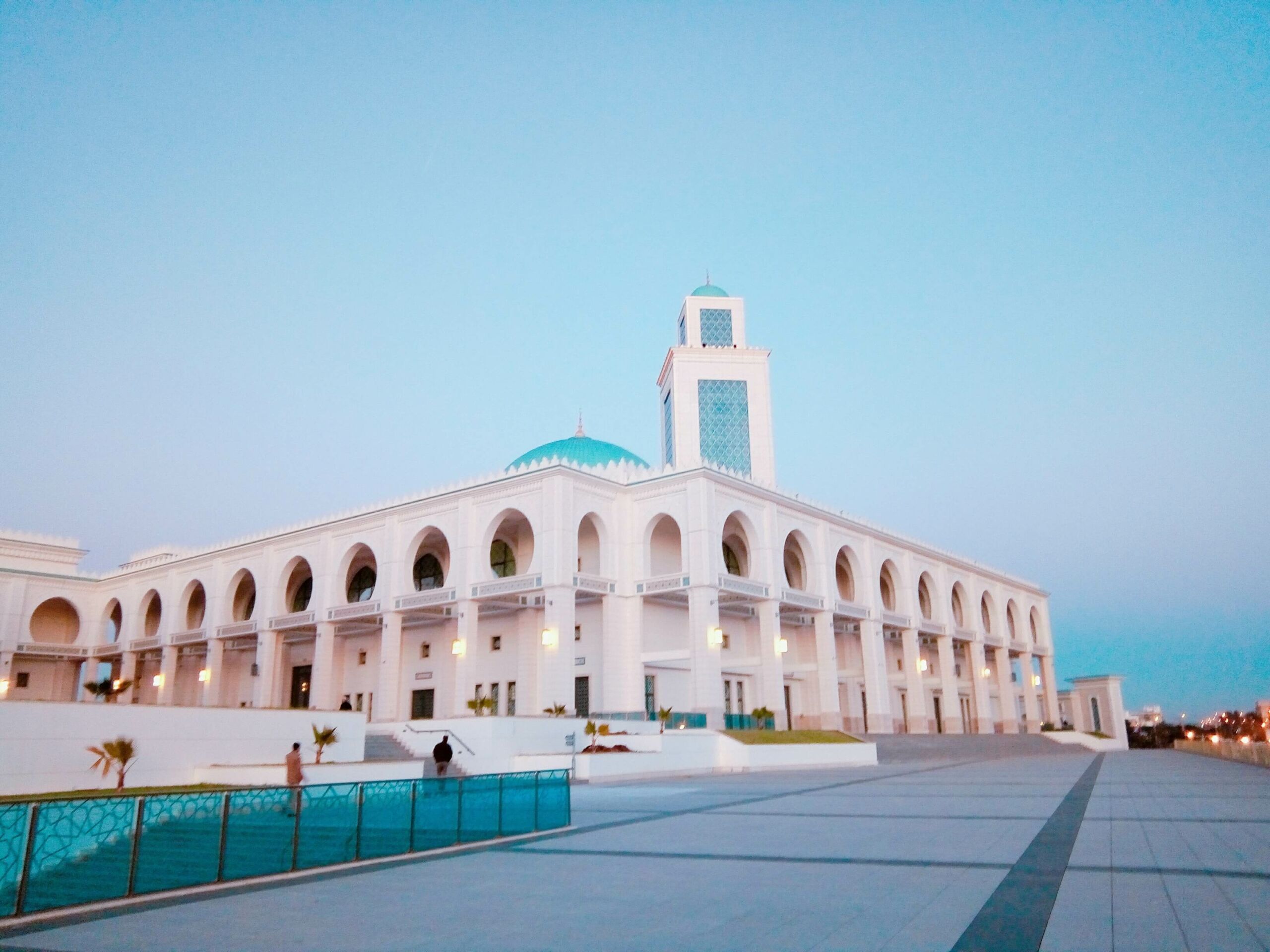
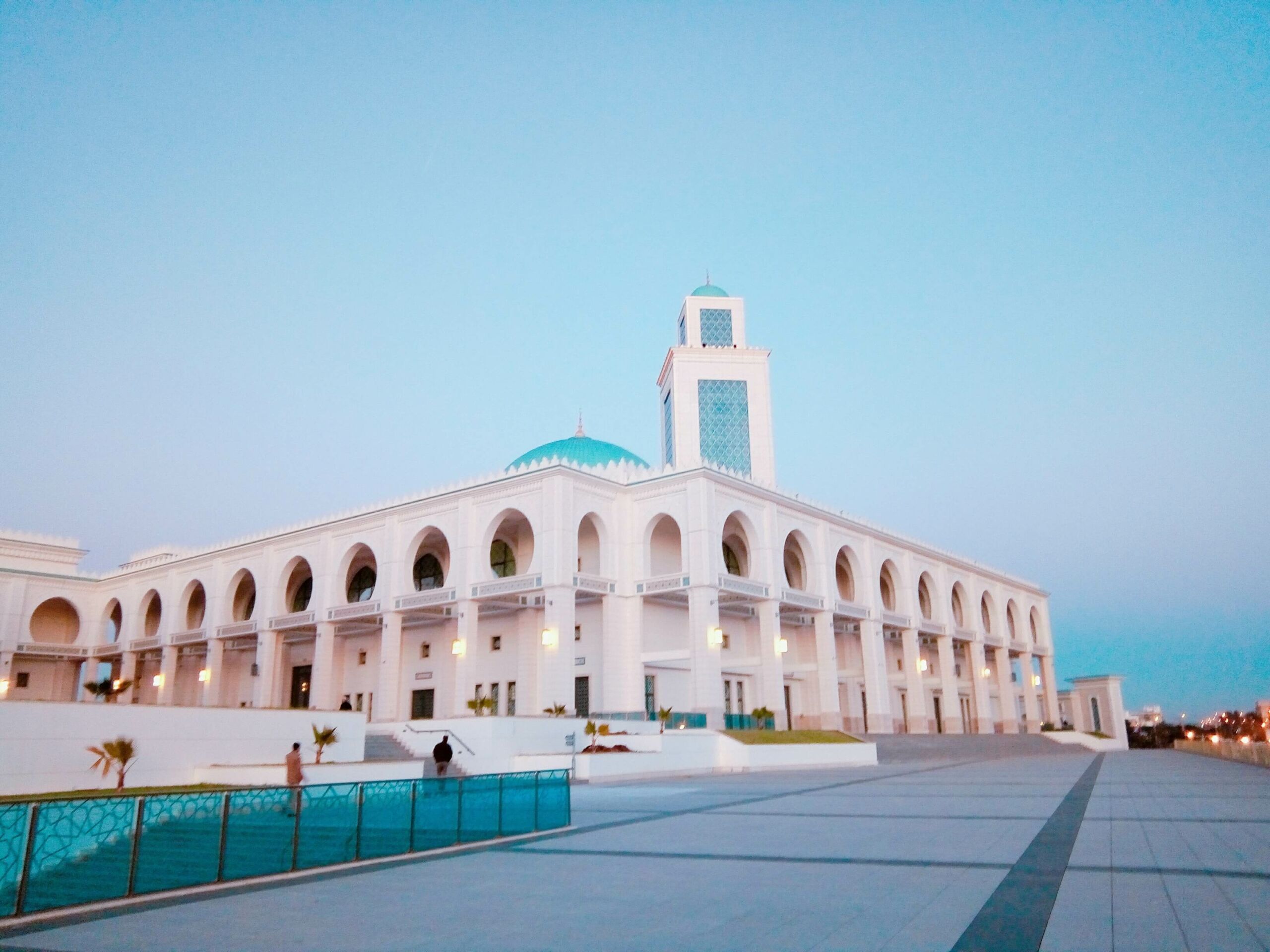
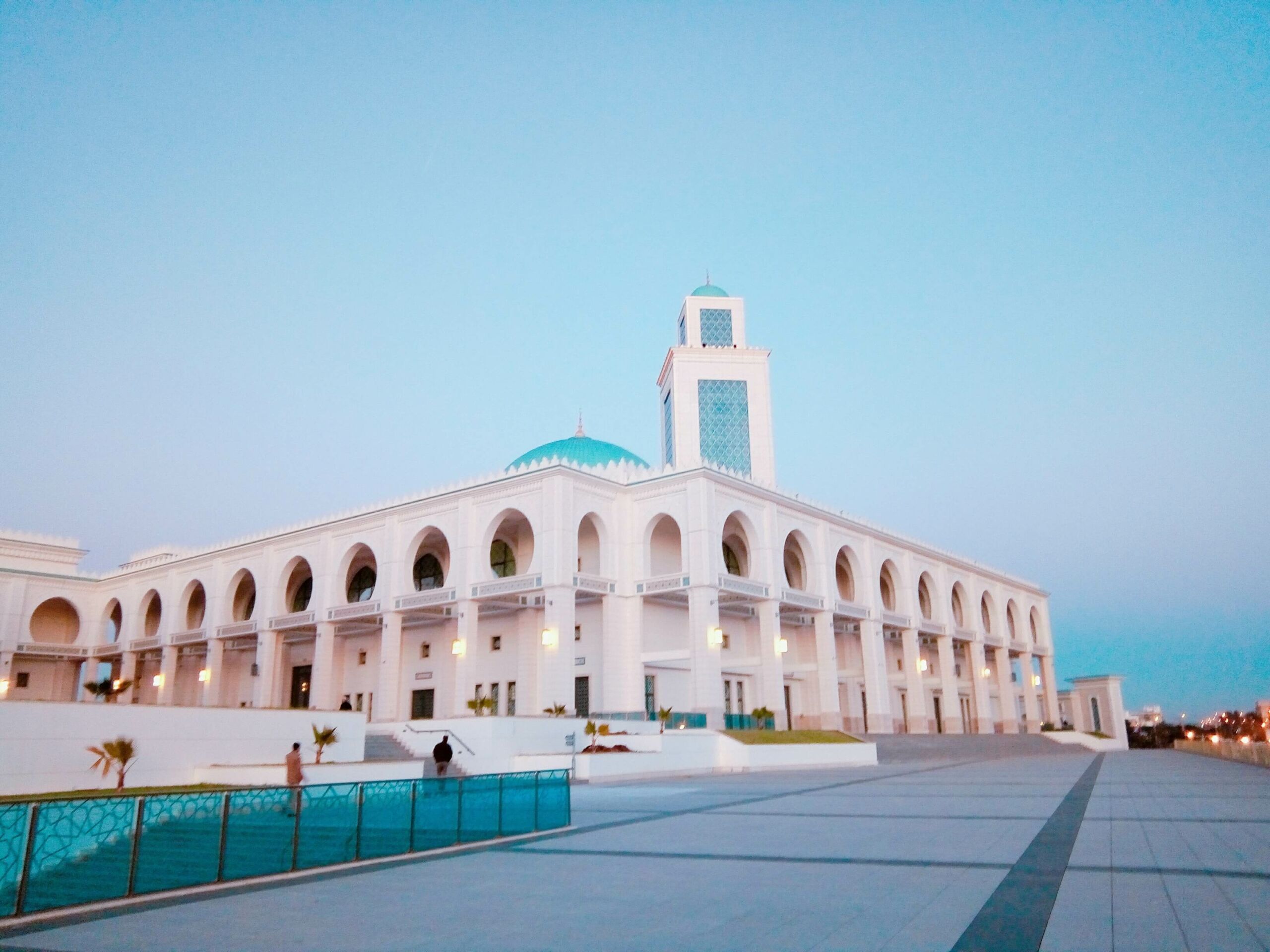
The position Property
The position property specifies the type of positioning method used for an element.
There are five different position values:
- static: are not affected by the top, bottom, left, and right properties.
- relative: the top, right, bottom, and left properties of a relatively-positioned element will cause it to be adjusted away from its normal position.
- fixed: positioned relative to the viewport, which means it always stays in the same place even if the page is scrolled.
- absolute: is positioned relative to the nearest positioned ancestor
- sticky: is positioned based on the user's scroll position.
I. Backgrounds
The background property is a shorthand for eight properties:
- background-image: Specifies an image from a file or a generated color gradient.
- background-position: Sets the initial position of the background image.
- background-size: Specifies how large to render the background image within the element.
- background-repeat: Determines whether to tile the image if necessary to fill the entire element.
- background-origin: Determines whether background positioning is relative to the element's border-box, padding-box (initial value), or content-box.
- background-clip: Specifies whether the background should fill the element's border-box (initial value), padding-box, or content-box.
- background-attachment: Specifies whether the background image will scroll up and down along with the element (the initial value), or if the image will be fixed in place in the viewport.
- background-color: Specifies a solid background color.
Also, using the shorthand property (background) will set the values you specify while also resetting all the others to their initial value.
The background-image property is particularly interesting. It accepts a path to an image URL (background-image: url(Oran.jpg)), but it can also accept a gradient function (see example below).
HTML
<div class="Gradient_Div" > </div >
CSS
.Gradient_Div {
background-image: linear-gradient(90deg, #00dbde, #fc00ff);
}
/* Using color stops */
.Gradient_Div {
background-image: linear-gradient(to right, #017137, #ffffff, #f47639);
}
/* Stripes */
.Gradient_Div {
background-image: linear-gradient( to bottom, #000000 0%, #000000 33%, #c71231 33%, #c71231 66%, #ffba32 66% );
}
/* Repeating gradients */
.Gradient_Div {
background-image: repeating-linear-gradient( to bottom, #be0b31 0%, #be0b31 10%, #ffffff 10%, #ffffff 20% );
}
Result
1. Linear gradient
The linear-gradient function
The linear-gradient function has three parameters defining its behavior: angle, starting color, and ending
color.
The angle of the gradient can be specified in several ways.
- Using words like: to right, to left, to top or to bottom. You can even specify a corner such as to bottom right
- You can use more specific units, such as degrees. The value 0deg points straight up (equivalent to to top); higher values move clockwise around the circle, so 90deg points to the right, 180deg points down, 360deg points up again.
- rad: indicates radians. One full circle is 2 π, or roughly 6.2832 radians.
- turn: indicates the number of full turns around the circle. For example, 0.25turn, is equal to 90deg.
- grad: indicates gradians. One full circle is 400 gradians (400grad) and 100grad equals 90deg.
Color stops
You can define a gradient with multiple colors, which are each called a color stop (see third row in the
example below).
The linear-gradient function can accept any number of color stops, each separated by a comma.
You can adjust the position of the color stops however you want. They also do not need to be measured in
percentages. Pixels, ems, and other length units are perfectly valid.
Stripes
If you place two color stops at the same position, the gradient's color will instantly switch from one to the
other, rather than a smooth transition (see forth row in the example below).
In the example above, the color #000000 and #c71231 have the same color stop which is 33%. In this case the
transition will be instant, creating a stripe effect.
Repeating gradients
We can use the repeating-linear-gradient function to create a gradient that repeats itself.
We use either color stops or stripes to create a pattern first then it will be repeated.
It can be used to create a progress bar, for example. (This effect requires a bit of JavaScript, which we will
cover in detai in Lab 5. If you to take a look at it now, go to Lab JS
code.)
You can find many websites that provide some aesthetic backgrounds with their CSS code. For example, this one.
2. Radial gradient
Radial gradients start at a single point and proceed outward in all directions.
By default, the gradient is centered in the element, transitioning evenly to its corners.
It's elliptical in shape, matching the proportions of the element (that is, wider for wide elements, taller
for tall elements).
Radial gradients support color stops, the same as linear gradients.
You can also make the radial gradient a circle rather than an ellipse, or you can specify where the gradient
should be centered.
A repeating-radialgradient() function repeats the pattern in concentric rings.
HTML
<div class="Gradient_Div" > </div >
CSS
.Gradient_Div {
background-image: radial-gradient(white, blue);
}
.Gradient_Div {
background-image: radial-gradient(circle, white, midnightblue)
}
.Gradient_Div {
/* Sized 3 em, centered 25% from the left and top edges */
background-image: radial-gradient(3em at 25% 25%, white, midnightblue);
}
.Gradient_Div {
/* Radial gradient with explicit color stop positions */
background-image: radial-gradient(circle, midnightblue 0%, white 75%, red 100%);
}
.Gradient_Div {
/* Repeating gradient with stripes */
background-image: repeating-radial-gradient(circle, midnightblue 0, midnightblue 1em, white 1em, white 2em);
}
Result
II. Shadows
Two properties that create shadows are box-shadow, which creates a shadow of an element's box shape, and
text-shadow, which creates a shadow of rendered text.
Shadows rules have the following syntax:
box-shadow: offset-x offset-y blur-radius spread-radius color;
- offset-x offset-y: how far the shadow will be shifted from the element's position (horizontal, then vertical). If these have a value of 0, then the shadow will be directly behind the element.
- blur-radius: controls how much the edges of the shadow are to be blurred. This will give it a softer, slightly transparent edge.
- spread-radius: The spread radius controls the size of the shadow. A positive spread radius makes the shadow larger in all directions; a negative value makes the shadow smaller.
- color: any color format.
- inset: makes the shadow appear inside the border of the element, rather than outside.
HTML
<div class="Shadow_Div" > </div >
CSS
.Shadow_Div {
/* Dark blue shadow with a 0.5 em blur */
box-shadow: 0.1em 0.1em 0.5em #124;
text-shadow: 0.1em 0.1em 0.5em #ffffff;
}
.Shadow_Div:active {/* When I click on it */
/* Several shadows definition can be added, separating them with a comma.*/
box-shadow: inset 0 0 0.5em #124, inset 0 0.5em 1em rgba(0, 0, 0, 0.4);
}
.Shadow_Div:hover {
box-shadow: 0 0 50px 15px #48abe0;
}
.Shadow_Div:hover {
box-shadow: 0 0 10px 5px #48abe0, 0 0 20px 7px #ebecca, 0 0 25px 20px #8a2be2, 0 0 30px 25px #ff1493;
}
.Shadow_Div:hover {
box-shadow: -10px -10px 0 5px #000000;
}
.Shadow_Div:hover {
box-shadow: 10px 10px 0 5px #000000;
}
.Shadow_Div:hover {
box-shadow: 0 10px 0 5px #000000;
}
Result
Hover or click for the effect.
Lab 3: CSS Responsive design
The three key principles to responsive design:
- A mobile first approach to design. This means you build the mobile version before you construct the desktop layout.
- The @media at-rule. With this rule, you can tailor your styles for viewports of different sizes. This syntax (often called media queries) lets you write styles that only apply under certain conditions.
- The use of fluid layouts. This approach allows containers to scale to different sizes based on the width of the viewport.
The viewport meta tag: is an HTML tag that tells mobile devices you've intentionally designed for small
screens. Without it, a mobile browser assumes your page is not responsive, and it will attempt to emulate a
desktop browser.
<meta name="viewport" content="width=device-width, initial-scale=1">
The meta tag's content attribute indicates two things. First, it tells the browser to use the device width as
the assumed width when interpreting the CSS, instead of pretending to be a full size desktop browser. Second,
it uses initial-scale to set the zoom level at 100% when the page loads.
I. Media queries
Media queries: allow you to write a set of styles that only apply to the page under certain conditions.
Media queries use the @media at-rule to target devices that match a specified feature.
The @media rule is a conditional check that must be true for any of these styles to be applied to the page.
You can further refine a media query by joining the two clauses with the keyword and (second example). This
combined media query only targets devices that meet both criteria.
If you want a media query that targets one of multiple criteria, use a comma (third example). This example
targets both viewports 560px and narrower and those 1200px and wider.
CSS
@media (min-width: 560px) { ....}
@media (min-width: 560px) and (max-width: 1200px) { .... }
@media (max-width: 560px), (min-width: 1200px) { .... }
min-width targets devices with a viewport above a certain width, and max-width targets devices below a certain
width are each called a media feature.
min-width and max-width are the most common ones you will use by far. But you can also use a number of other
types of media features.
Here are some examples:
- (min-height: 500px): Targets viewports 500px and taller;
- (max-height: 500px): Targets viewports 500px and shorter
- (orientation: landscape): Targets viewports that are wider than they are tall;
- (orientation: portrait): Targets viewports that are taller than they are wide;
- (min-resolution: 2dppx): Targets devices with a screen resolution of 2 dots per pixel or higher; targets retina displays;
- (max-resolution: 2dppx): Targets devices with a screen resolution of up to 2 dots per pixel
TIP 1: You can also place a media query in the <link> tag. Adding <link rel="stylesheet" media="(min-width: 500px)" href="large-screen.css" /> to your page will apply the contents of the large-screen.css file to the page only if the min-width media query is true. Note that the stylesheet will always download, regardless of the width of the viewport, so this is merely a tactic for code organization, not network traffic reduction.
TIP 2: The two media types you will generally need to think about are screen and print. Using a print media query lets you control how your page will lay out if the user prints the page, so you can do things like removing background images (to save on ink) and hiding unneeded navigation. When a user prints the page, they typically only want the main page text.
To write print styles that apply only when printing, use the query @media print. To target screen only, use
@media screen.
Always be sure each media query comes after the styles it overrides, so the styles within the media query take
precedence.
II. Responsive images
The best practice is to create a few copies of an image, each at a different resolution.
Use the srcset attribute (short for “source set”). This attribute is a newer addition to HTML. It allows you
to specify multiple image URLs for one <img> tag, specifying the resolution of
each. The browser will then figure out which image it needs and download that one.
Most browsers now support srcset, but those that don't will fall back to the specified src, loading whichever
URL is specified there. This
HTML
<img alt="Oran-pic"
src="IMAGES/Oran.jpg"
srcset="IMAGES/Oran-small.jpg 560w,
IMAGES/Oran-medium.jpg 800w,
IMAGES/Oran-big.jpg 1280w"
/>
III. Example
In this example, we will create a responsive navigation bar.
The navigation bar will have a logo on the right, and menu items on the left. When the viewport is wide, the
menu items will be displayed horizontally.
The menu items will be displayed horizontally when the viewport is large.
When the viewport is narrow, the menu items will be hidden (right: -100%; opacity: 0;) and a burger menu icon
will appear instead (.Menu_Icon {display: block;...).
When the user clicks on the burger menu icon, the menu items will appear (.Nav_Bar_Menu {right: 0; opacity:
1;...).
The clicking on the burger menu icon need to be handled by JavaScript. You can find the code here.
HTML
<nav class="Nav_Bar_Items">
<h1 class="Nav_Bar_Logo">سياحة</h1>
<div class="Menu_Icon" id="Menu_Icon_ID">
<i class="fas fa-bars" id="Burger_Icon_ID"> </i>
</div>
<ul class="Nav_Bar_Menu" id="Nav_Bar_Menu_ID">
<li>
<a href="#" class="Nav_Bar_Menu_Item">
<span>إتصل بنا</span>
<i class="fa-solid fa-envelope"> </i>
</a>
</li>
<li>
<a href="#" class="Nav_Bar_Menu_Item">
<span>جميع الخدمات</span>
<i class="fa-solid fa-briefcase"> </i>
</a>
</li>
<li>
<a href="#" class="Nav_Bar_Menu_Item">
<span>من نحن؟</span>
<i class="fa-solid fa-circle-info"> </i>
</a>
</li>
<li>
<a href="#" class="Nav_Bar_Menu_Item">
<span>الرئيسية</span>
<i class="fa-solid fa-house"> </i>
</a>
</li>
</ul>
</nav>
CSS
@media screen and (max-width: 850px) {
Nav_Bar_Menu {
display: flex;
flex-direction: column;
right: -100%;
opacity: 0;
}
.Menu_Icon {
display: block;
cursor: pointer;
}
.Nav_Bar_Menu.active {
right: 0;
opacity: 1;
}
Result
You can find the CSS code of the responsiveness for this example, here.